如何在c++中实现双向链表
时间: 2024-02-23 09:59:03 浏览: 20
在C++中实现双向链表,可以按照以下步骤:
1. 定义链表节点的结构体,包括数据成员和指向前一个和后一个节点的指针。
```c++
struct ListNode {
int val;
ListNode *prev;
ListNode *next;
ListNode(int x) : val(x), prev(nullptr), next(nullptr) {}
};
```
2. 定义双向链表类,包括头节点和尾节点指针,以及一些基本的操作方法,如插入节点、删除节点、查找节点等。
```c++
class DoublyLinkedList {
public:
DoublyLinkedList() : head(nullptr), tail(nullptr) {}
void insertFront(int val) {
ListNode* newNode = new ListNode(val);
if (!head) {
head = tail = newNode;
} else {
head->prev = newNode;
newNode->next = head;
head = newNode;
}
}
void insertBack(int val) {
ListNode* newNode = new ListNode(val);
if (!tail) {
head = tail = newNode;
} else {
tail->next = newNode;
newNode->prev = tail;
tail = newNode;
}
}
void deleteNode(int val) {
ListNode* cur = head;
while (cur) {
if (cur->val == val) {
if (cur == head) {
head = cur->next;
if (head) head->prev = nullptr;
} else if (cur == tail) {
tail = cur->prev;
if (tail) tail->next = nullptr;
} else {
cur->prev->next = cur->next;
cur->next->prev = cur->prev;
}
delete cur;
break;
}
cur = cur->next;
}
}
ListNode* findNode(int val) {
ListNode* cur = head;
while (cur) {
if (cur->val == val) return cur;
cur = cur->next;
}
return nullptr;
}
private:
ListNode *head;
ListNode *tail;
};
```
这样就可以通过调用双向链表的方法来实现插入、删除、查找等功能了。
相关推荐
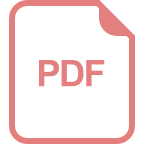
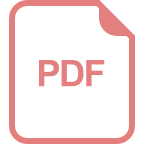
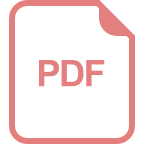
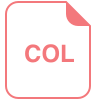
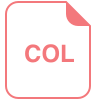
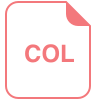
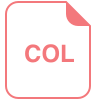
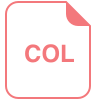









