c++定义一个双向链表
时间: 2023-11-03 13:01:20 浏览: 98
在C语言中,可以通过定义一个结构体来实现双向链表。结构体应包含一个数据成员和两个指向前驱和后继节点的指针。下面是一个示例代码:
```c
typedef struct _DOUBLE_LINK_NODE {
int data;
struct _DOUBLE_LINK_NODE* prev;
struct _DOUBLE_LINK_NODE* next;
} DOUBLE_LINK_NODE;
```
以上代码定义了一个名为`DOUBLE_LINK_NODE`的结构体,包含一个整型数据成员`data`和两个指向前驱和后继节点的指针`prev`和`next`。
相关问题
用C++写一段代码定义一个双向链表类以及其常用功能
在C++中,我们可以定义一个双向链表类(Doubly Linked List),包含节点结构体、头指针和一些基本操作函数,如插入、删除、遍历等。以下是简单的代码示例:
```cpp
#include <iostream>
// 定义链表节点结构
struct Node {
int data;
Node* prev;
Node* next;
};
class DoublyLinkedList {
public:
// 构造函数,初始化空链表
DoublyLinkedList() : head(nullptr), tail(nullptr) {}
// 插入节点到链表头部
void insertAtStart(int value) {
Node* newNode = new Node{value, nullptr, head};
if (head) head->prev = newNode;
head = newNode;
if (!tail) tail = newNode;
}
// 插入节点到链表尾部
void insertAtEnd(int value) {
Node* newNode = new Node{value, tail, nullptr};
if (tail) tail->next = newNode;
tail = newNode;
if (!head) head = newNode;
}
// 删除指定值的节点
void removeNode(int value) {
Node* curr = head;
while (curr) {
if (curr->data == value) {
if (curr->prev) curr->prev->next = curr->next;
else head = curr->next;
if (curr->next) curr->next->prev = curr->prev;
else tail = curr->prev;
delete curr;
return;
}
curr = curr->next;
}
}
// 遍历链表并打印元素
void display() {
Node* temp = head;
while (temp) {
std::cout << temp->data << " ";
temp = temp->next;
}
std::cout << "\n";
}
private:
Node* head; // 链表头节点
Node* tail; // 链表尾节点
};
int main() {
DoublyLinkedList list;
list.insertAtStart(5);
list.insertAtStart(3);
list.insertAtEnd(7);
list.display();
list.removeNode(5);
list.display();
return 0;
}
```
写一个C++程序:定义一个双向链表,并给出其增删改查的函数
好的,以下是所需的C语言程序:
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* prev;
struct Node* next;
} Node;
typedef struct List {
Node* head;
Node* tail;
int size;
} List;
void init(List* list) {
list->head = NULL;
list->tail = NULL;
list->size = 0;
}
void add(List* list, int data) {
Node* node = (Node*)malloc(sizeof(Node));
node->data = data;
node->prev = list->tail;
node->next = NULL;
if (list->tail != NULL) {
list->tail->next = node;
}
list->tail = node;
if (list->head == NULL) {
list->head = node;
}
list->size++;
}
void remove_last(List* list) {
if (list->size > 0) {
Node* node = list->tail;
if (list->tail == list->head) {
list->tail = NULL;
list->head = NULL;
} else {
list->tail = node->prev;
node->prev->next = NULL;
}
free(node);
list->size--;
}
}
void update(List* list, int index, int data) {
if (index < list->size) {
Node* node = list->head;
for (int i = 0; i < index; i++) {
node = node->next;
}
node->data = data;
}
}
int find(List* list, int data) {
Node* node = list->head;
int index = 0;
while (node != NULL) {
if (node->data == data) {
return index;
}
index++;
node = node->next;
}
return -1;
}
int main() {
List list;
init(&list);
add(&list, 1);
add(&list, 2);
add(&list, 3);
add(&list, 4);
remove_last(&list);
update(&list, 1, 5);
printf("The index of data 3 is %d\n", find(&list, 3));
printf("The index of data 5 is %d\n", find(&list, 5));
return 0;
}
回答完毕。
阅读全文
相关推荐
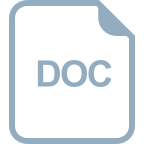
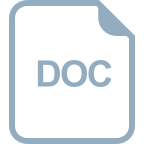
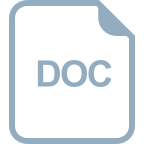
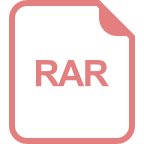
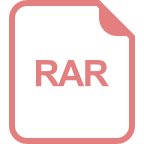
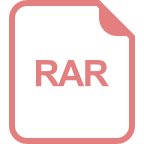
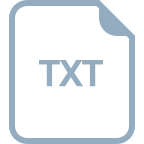
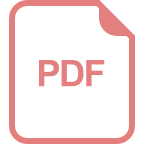
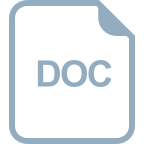
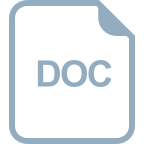





