用c++写一个双向循环链表的输入和打印
时间: 2024-10-27 15:10:38 浏览: 22
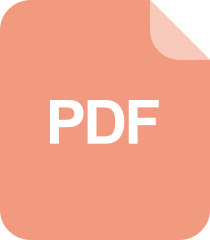
如何用C++实现双向循环链表
在C++中,双向循环链表是一种特殊的链表结构,其中每个节点包含两个指针,分别指向前一个和后一个节点,形成一个无限循环。以下是创建一个双向循环链表、插入元素、以及打印链表的基本步骤:
```cpp
#include <iostream>
// 定义双向链表节点结构体
struct Node {
int data;
Node* prev; // 指向前一个节点
Node* next; // 指向后一个节点
};
// 初始化双向循环链表为空
Node* createCircularList() {
return nullptr;
}
// 插入新节点到链表
void insert(Node*& head, int value) {
if (head == nullptr) {
head = new Node();
head->data = value;
head->prev = head;
head->next = head;
} else {
Node* newNode = new Node();
newNode->data = value;
newNode->prev = head->prev;
newNode->next = head;
head->prev->next = newNode;
head->prev = newNode;
}
}
// 打印链表(包括环)
void printCircularList(Node* head) {
if (head != nullptr) {
Node* temp = head;
do {
std::cout << temp->data << " ";
temp = temp->next;
} while (temp != head);
std::cout << "..." << std::endl;
} else {
std::cout << "链表为空" << std::endl;
}
}
int main() {
Node* listHead = createCircularList();
// 插入一些数据示例
for (int i = 1; i <= 5; ++i) {
insert(listHead, i);
}
// 打印链表
printCircularList(listHead);
return 0;
}
```
阅读全文
相关推荐
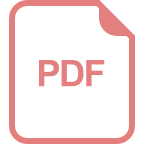
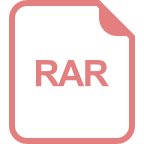
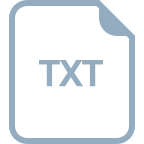
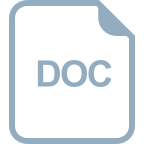









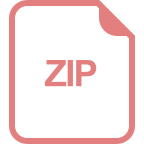