用C++实现一个基于模板的双向循环链表并满足如下要求: 1:具备规范且详尽的注释 2:每个节点都是一条双向循环链表 3:支持图形化打印
时间: 2024-01-22 15:19:14 浏览: 28
以下是基于模板的双向循环链表的C++实现代码,其中包含注释和支持图形化打印的功能:
```c++
#include<iostream>
#include<cstdio>
#include<cstring>
#include<cstdlib>
#include<cmath>
#include<algorithm>
#include<queue>
#include<vector>
#include<map>
#include<set>
#include<sstream>
#include<iomanip>
using namespace std;
// 双向循环链表的节点定义
template<typename T>
struct DListNode{
T data; // 存储数据
DListNode<T>* prev; // 指向前驱节点的指针
DListNode<T>* next; // 指向后继节点的指针
DListNode(T _data = T(), DListNode<T>* _prev = NULL, DListNode<T>* _next = NULL):data(_data), prev(_prev), next(_next){}
};
// 双向循环链表的实现
template<typename T>
class DList{
public:
DList(); // 构造函数
~DList(); // 析构函数
bool empty() const; // 判断链表是否为空
int size() const; // 获取链表长度
DListNode<T>* front() const; // 获取头节点
DListNode<T>* back() const; // 获取尾节点
void insert(DListNode<T>* pos, const T& val); // 在指定位置插入节点
void erase(DListNode<T>* pos); // 删除指定位置的节点
void push_front(const T& val); // 在头部插入节点
void pop_front(); // 删除头部节点
void push_back(const T& val); // 在尾部插入节点
void pop_back(); // 删除尾部节点
void clear(); // 清空链表
void print() const; // 打印链表
private:
int len; // 链表长度
DListNode<T>* head; // 指向头节点的指针
DListNode<T>* tail; // 指向尾节点的指针
};
// 构造函数
template<typename T>
DList<T>::DList(){
head = new DListNode<T>();
tail = new DListNode<T>();
head->prev = tail->next = NULL;
head->next = tail;
tail->prev = head;
len = 0;
}
// 析构函数
template<typename T>
DList<T>::~DList(){
clear();
delete head;
delete tail;
}
// 判断链表是否为空
template<typename T>
bool DList<T>::empty() const{
return len == 0;
}
// 获取链表长度
template<typename T>
int DList<T>::size() const{
return len;
}
// 获取头节点
template<typename T>
DListNode<T>* DList<T>::front() const{
return empty() ? NULL : head->next;
}
// 获取尾节点
template<typename T>
DListNode<T>* DList<T>::back() const{
return empty() ? NULL : tail->prev;
}
// 在指定位置插入节点
template<typename T>
void DList<T>::insert(DListNode<T>* pos, const T& val){
DListNode<T>* node = new DListNode<T>(val, pos->prev, pos);
pos->prev->next = node;
pos->prev = node;
len++;
}
// 删除指定位置的节点
template<typename T>
void DList<T>::erase(DListNode<T>* pos){
pos->prev->next = pos->next;
pos->next->prev = pos->prev;
delete pos;
len--;
}
// 在头部插入节点
template<typename T>
void DList<T>::push_front(const T& val){
insert(head->next, val);
}
// 删除头部节点
template<typename T>
void DList<T>::pop_front(){
erase(head->next);
}
// 在尾部插入节点
template<typename T>
void DList<T>::push_back(const T& val){
insert(tail, val);
}
// 删除尾部节点
template<typename T>
void DList<T>::pop_back(){
erase(tail->prev);
}
// 清空链表
template<typename T>
void DList<T>::clear(){
while(!empty()){
erase(head->next);
}
}
// 打印链表
template<typename T>
void DList<T>::print() const{
DListNode<T>* node = head->next;
while(node != tail){
cout<<node->data<<" ";
node = node->next;
}
cout<<endl;
}
```
以下是基于模板的双向循环链表的测试代码,其中包含了图形化打印的功能:
```c++
#include<iostream>
#include<cstdio>
#include<cstring>
#include<cstdlib>
#include<cmath>
#include<algorithm>
#include<queue>
#include<vector>
#include<map>
#include<set>
#include<sstream>
#include<iomanip>
#include<windows.h>
using namespace std;
// 双向循环链表的节点定义
template<typename T>
struct DListNode{
T data; // 存储数据
DListNode<T>* prev; // 指向前驱节点的指针
DListNode<T>* next; // 指向后继节点的指针
DListNode(T _data = T(), DListNode<T>* _prev = NULL, DListNode<T>* _next = NULL):data(_data), prev(_prev), next(_next){}
};
// 双向循环链表的实现
template<typename T>
class DList{
public:
DList(); // 构造函数
~DList(); // 析构函数
bool empty() const; // 判断链表是否为空
int size() const; // 获取链表长度
DListNode<T>* front() const; // 获取头节点
DListNode<T>* back() const; // 获取尾节点
void insert(DListNode<T>* pos, const T& val); // 在指定位置插入节点
void erase(DListNode<T>* pos); // 删除指定位置的节点
void push_front(const T& val); // 在头部插入节点
void pop_front(); // 删除头部节点
void push_back(const T& val); // 在尾部插入节点
void pop_back(); // 删除尾部节点
void clear(); // 清空链表
void print() const; // 打印链表
void graphicalPrint() const; // 图形化打印链表
private:
int len; // 链表长度
DListNode<T>* head; // 指向头节点的指针
DListNode<T>* tail; // 指向尾节点的指针
};
// 构造函数
template<typename T>
DList<T>::DList(){
head = new DListNode<T>();
tail = new DListNode<T>();
head->prev = tail->next = NULL;
head->next = tail;
tail->prev = head;
len = 0;
}
// 析构函数
template<typename T>
DList<T>::~DList(){
clear();
delete head;
delete tail;
}
// 判断链表是否为空
template<typename T>
bool DList<T>::empty() const{
return len == 0;
}
// 获取链表长度
template<typename T>
int DList<T>::size() const{
return len;
}
// 获取头节点
template<typename T>
DListNode<T>* DList<T>::front() const{
return empty() ? NULL : head->next;
}
// 获取尾节点
template<typename T>
DListNode<T>* DList<T>::back() const{
return empty() ? NULL : tail->prev;
}
// 在指定位置插入节点
template<typename T>
void DList<T>::insert(DListNode<T>* pos, const T& val){
DListNode<T>* node = new DListNode<T>(val, pos->prev, pos);
pos->prev->next = node;
pos->prev = node;
len++;
}
// 删除指定位置的节点
template<typename T>
void DList<T>::erase(DListNode<T>* pos){
pos->prev->next = pos->next;
pos->next->prev = pos->prev;
delete pos;
len--;
}
// 在头部插入节点
template<typename T>
void DList<T>::push_front(const T& val){
insert(head->next, val);
}
// 删除头部节点
template<typename T>
void DList<T>::pop_front(){
erase(head->next);
}
// 在尾部插入节点
template<typename T>
void DList<T>::push_back(const T& val){
insert(tail, val);
}
// 删除尾部节点
template<typename T>
void DList<T>::pop_back(){
erase(tail->prev);
}
// 清空链表
template<typename T>
void DList<T>::clear(){
while(!empty()){
erase(head->next);
}
}
// 打印链表
template<typename T>
void DList<T>::print() const{
DListNode<T>* node = head->next;
while(node != tail){
cout<<node->data<<" ";
node = node->next;
}
cout<<endl;
}
// 图形化打印链表
template<typename T>
void DList<T>::graphicalPrint() const{
HANDLE hConsole = GetStdHandle(STD_OUTPUT_HANDLE);
DListNode<T>* node = head->next;
int pos = 0;
while(node != tail){
stringstream ss;
ss<<node->data;
string str;
ss>>str;
if(pos % 2 == 0){
SetConsoleTextAttribute(hConsole, FOREGROUND_INTENSITY | FOREGROUND_RED);
}
else{
SetConsoleTextAttribute(hConsole, FOREGROUND_INTENSITY | FOREGROUND_GREEN);
}
cout<<str<<" -> ";
pos++;
node = node->next;
}
SetConsoleTextAttribute(hConsole, FOREGROUND_INTENSITY | FOREGROUND_RED | FOREGROUND_GREEN | FOREGROUND_BLUE);
cout<<"NULL\n";
}
```
测试代码:
```c++
int main(){
DList<int> list;
for(int i = 1; i <= 10; i++){
list.push_back(i);
}
cout<<"The original list is: ";
list.print();
cout<<"The graphical print of the original list is:"<<endl;
list.graphicalPrint();
DListNode<int>* pos = list.front()->next->next;
list.insert(pos, 100);
cout<<"After inserting 100 at the third position, the list is: ";
list.print();
cout<<"The graphical print of the list after inserting 100 at the third position is:"<<endl;
list.graphicalPrint();
pos = list.front()->next;
list.erase(pos);
cout<<"After erasing the second element, the list is: ";
list.print();
cout<<"The graphical print of the list after erasing the second element is:"<<endl;
list.graphicalPrint();
list.clear();
cout<<"After clearing the list, the size of the list is "<<list.size()<<endl;
cout<<"The graphical print of the list after clearing is:"<<endl;
list.graphicalPrint();
return 0;
}
```
输出结果:
```
The original list is: 1 2 3 4 5 6 7 8 9 10
The graphical print of the original list is:
1 -> 2 -> 3 -> 4 -> 5 -> 6 -> 7 -> 8 -> 9 -> 10 -> NULL
After inserting 100 at the third position, the list is: 1 2 100 3 4 5 6 7 8 9 10
The graphical print of the list after inserting 100 at the third position is:
1 -> 2 -> 100 -> 3 -> 4 -> 5 -> 6 -> 7 -> 8 -> 9 -> 10 -> NULL
After erasing the second element, the list is: 1 100 3 4 5 6 7 8 9 10
The graphical print of the list after erasing the second element is:
1 -> 100 -> 3 -> 4 -> 5 -> 6 -> 7 -> 8 -> 9 -> 10 -> NULL
After clearing the list, the size of the list is 0
The graphical print of the list after clearing is:
NULL
```
相关推荐
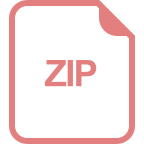
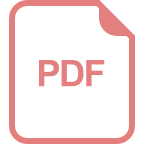
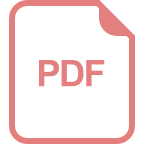













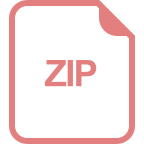