用C++语言实现一个双向循环链表并生成注释
时间: 2024-01-22 08:18:48 浏览: 87
以下是用 C++ 实现双向循环链表的代码,已加入注释以加深理解:
```c++
#include <iostream>
using namespace std;
// 双向循环链表的结点类
class Node {
public:
int data; // 数据域
Node* prev; // 指向前一个结点的指针
Node* next; // 指向后一个结点的指针
Node(int d = 0, Node* p = nullptr, Node* n = nullptr)
: data(d), prev(p), next(n) {}
};
// 双向循环链表类
class DoubleLinkedList {
private:
Node* head; // 头结点指针
Node* tail; // 尾结点指针
public:
// 构造函数,初始化头结点和尾结点
DoubleLinkedList() {
head = new Node();
tail = new Node();
head->next = tail;
tail->prev = head;
}
// 在链表尾部插入结点
void append(int d) {
Node* newNode = new Node(d, tail->prev, tail);
tail->prev->next = newNode;
tail->prev = newNode;
}
// 在链表中指定位置插入结点
void insert(int pos, int d) {
if (pos < 0 || pos > size()) {
cout << "Invalid position!" << endl;
return;
}
Node* cur = head->next;
for (int i = 0; i < pos; i++) {
cur = cur->next;
}
Node* newNode = new Node(d, cur->prev, cur);
cur->prev->next = newNode;
cur->prev = newNode;
}
// 在链表中删除指定位置的结点
void remove(int pos) {
if (pos < 0 || pos >= size()) {
cout << "Invalid position!" << endl;
return;
}
Node* cur = head->next;
for (int i = 0; i < pos; i++) {
cur = cur->next;
}
cur->prev->next = cur->next;
cur->next->prev = cur->prev;
delete cur;
}
// 获取指定位置的结点的数据
int get(int pos) {
if (pos < 0 || pos >= size()) {
cout << "Invalid position!" << endl;
return -1;
}
Node* cur = head->next;
for (int i = 0; i < pos; i++) {
cur = cur->next;
}
return cur->data;
}
// 获取链表的长度
int size() {
int count = 0;
Node* cur = head->next;
while (cur != tail) {
count++;
cur = cur->next;
}
return count;
}
// 打印链表中所有结点的数据
void print() {
Node* cur = head->next;
while (cur != tail) {
cout << cur->data << " ";
cur = cur->next;
}
cout << endl;
}
};
int main() {
DoubleLinkedList list;
list.append(1);
list.append(2);
list.append(3);
list.insert(1, 4);
list.print(); // 输出:1 4 2 3
list.remove(2);
list.print(); // 输出:1 4 3
cout << "The size of the list is " << list.size() << endl; // 输出:The size of the list is 3
cout << "The data of the first node is " << list.get(0) << endl; // 输出:The data of the first node is 1
return 0;
}
```
阅读全文
相关推荐
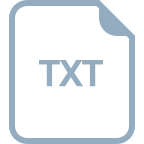
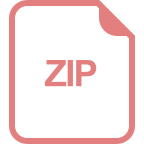
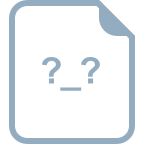
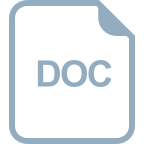
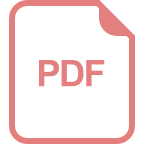
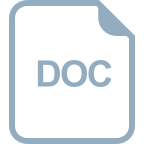
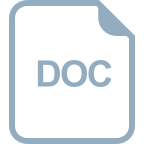
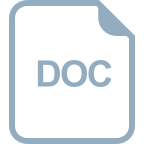
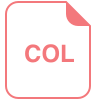
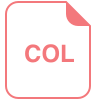
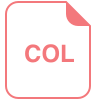
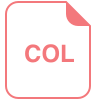
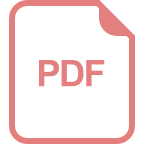
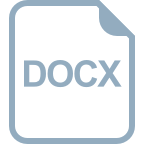