c++编程,根据一个单向链表,复制建立一个双向链表,然后输出双向链表。
时间: 2024-10-17 20:05:36 浏览: 28
在C++中,将单向链表转换成双向链表通常涉及以下几个步骤:
1. 定义结构体或类:首先,你需要定义一个双向链表节点(Node),它包含数据域(data)、指向前驱节点的指针(prev)以及指向后继节点的指针(next)。
```cpp
struct Node {
int data;
Node* prev;
Node* next;
};
```
2. 创建复制函数:创建一个接受单向链表头结点作为输入的函数,遍历单向链表,为每个节点创建对应的双向链表节点,并链接它们。
```cpp
Node* copySingleToDouble(Node* head) {
if (!head) return nullptr;
// 创建一个新的双向链表头节点
Node* newHead = new Node{head->data, nullptr, nullptr};
// 初始化新链表的前驱和后继指针
Node* current = newHead;
Node* newNode = nullptr;
// 遍历原链表
while (head) {
newNode = new Node{head->data, nullptr, nullptr};
newNode->next = current->next; // 将原节点的下一个节点赋给新节点
if (current->next) current->next->prev = newNode; // 更新新节点的前驱
current->next = newNode; // 新节点成为当前节点的后继
current = newNode; // 移动到新节点
head = head->next;
}
return newHead;
}
```
3. 输出双向链表:最后,你可以遍历新的双向链表并打印节点值。
```cpp
void printList(Node* node) {
while (node) {
cout << node->data << " ";
node = node->next;
}
cout << endl;
}
int main() {
// 原始单向链表操作...
Node* singleListHead = ...;
// 转换为双向链表
Node* doubleListHead = copySingleToDouble(singleListHead);
// 输出结果
printList(doubleListHead);
// 清理内存
delete doubleListHead;
return 0;
}
```
阅读全文
相关推荐
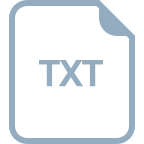
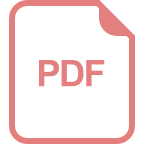
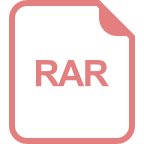
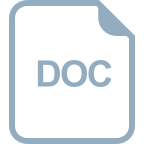






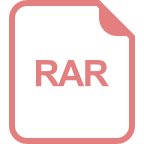
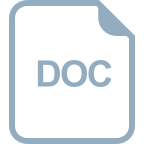






