编写C++程序建立一个单向链表。链表结点中的数据为从键盘输入的一个字符串,但要求将该串字符按由小到大的顺序组织在链表中。
时间: 2024-02-16 07:02:46 浏览: 81
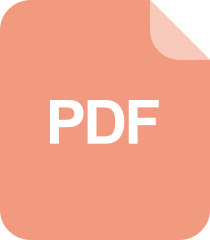
单向链表链表结点的插入-欧姆龙旋转编码器(技术篇)
好的,这是一个比较常见的链表问题。以下是一个简单的C++程序,可以建立一个单向链表,将用户从键盘输入的字符串按字母顺序插入链表中。
```c++
#include <iostream>
#include <string>
using namespace std;
class Node {
public:
string data;
Node* next;
};
class LinkedList {
public:
Node* head;
LinkedList() {
head = NULL;
}
void insert(string value) {
Node* newNode = new Node();
newNode->data = value;
newNode->next = NULL;
if (head == NULL) {
head = newNode;
return;
}
if (value < head->data) {
newNode->next = head;
head = newNode;
return;
}
Node* curr = head;
while (curr->next != NULL && curr->next->data < value) {
curr = curr->next;
}
newNode->next = curr->next;
curr->next = newNode;
}
void print() {
Node* curr = head;
while (curr != NULL) {
cout << curr->data << " ";
curr = curr->next;
}
cout << endl;
}
};
int main() {
LinkedList list;
string str;
while (cin >> str) {
list.insert(str);
}
list.print();
return 0;
}
```
该程序首先定义了两个类:Node 和 LinkedList。其中,Node 表示链表中的结点,包含一个字符串类型的数据成员和一个指向下一个结点的指针;LinkedList 表示一个单向链表,包含一个指向链表头的指针。程序中定义了链表的两个基本操作:插入和打印。在插入操作中,程序首先创建一个新的结点,将输入的字符串赋值给该结点的数据成员。然后,程序判断链表是否为空,如果为空,则将该结点设置为链表头;否则,程序遍历链表,找到第一个大于等于该结点值的位置,将该结点插入到这个位置之前。最后,程序调用 print() 函数打印链表中的所有结点。
你可以通过输入一些字符串来测试该程序,例如:
```
hello world this is a test program for linked list
```
程序会将这些字符串按字母顺序组织在链表中,并打印出来:
```
a for hello is list linked program test this world
```
阅读全文
相关推荐
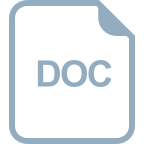
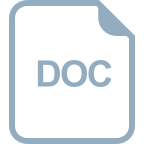


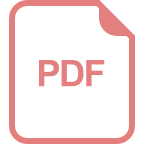
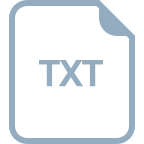
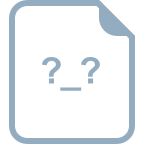
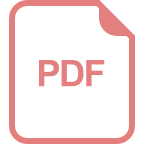
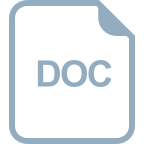
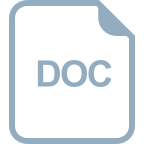
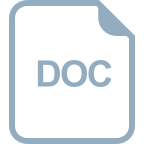
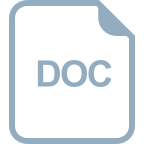
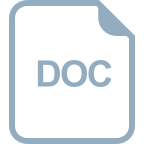
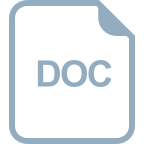
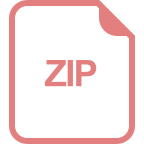
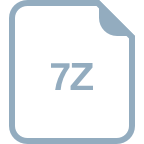
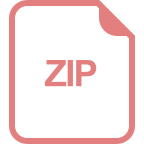
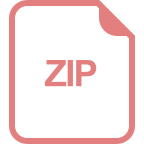
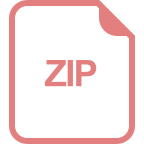