编写一个c程序,用fwrite将文件中的内容写到链表中,并查找链表中是否有键盘输入的字符串
时间: 2023-05-20 14:02:18 浏览: 102
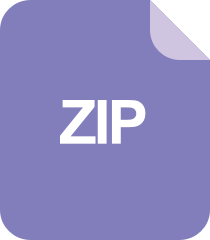
C语言链表实现字符串输入、查找、删除等
好的,以下是一个简单的C程序,它可以将文件中的内容写入链表,并查找链表中是否有键盘输入的字符串:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LEN 100
typedef struct node {
char data[MAX_LEN];
struct node *next;
} Node;
int main() {
FILE *fp;
char filename[MAX_LEN];
char input[MAX_LEN];
Node *head = NULL;
Node *current = NULL;
Node *prev = NULL;
printf("请输入文件名:");
scanf("%s", filename);
fp = fopen(filename, "r");
if (fp == NULL) {
printf("无法打开文件 %s\n", filename);
exit(1);
}
while (fgets(input, MAX_LEN, fp) != NULL) {
current = (Node *) malloc(sizeof(Node));
strcpy(current->data, input);
current->next = NULL;
if (head == NULL) {
head = current;
} else {
prev->next = current;
}
prev = current;
}
fclose(fp);
printf("请输入要查找的字符串:");
scanf("%s", input);
current = head;
while (current != NULL) {
if (strstr(current->data, input) != NULL) {
printf("找到了字符串 %s\n", input);
break;
}
current = current->next;
}
if (current == NULL) {
printf("没有找到字符串 %s\n", input);
}
return 0;
}
```
这个程序首先要求用户输入文件名,然后打开文件并逐行读取文件内容,将每一行内容存储在一个链表节点中。然后程序要求用户输入要查找的字符串,程序会遍历链表,查找是否有节点的数据包含该字符串。如果找到了,程序会输出一条消息,否则会输出另一条消息。
阅读全文
相关推荐
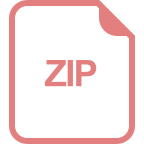


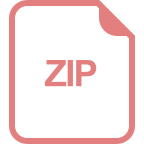
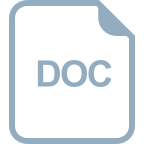



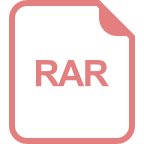
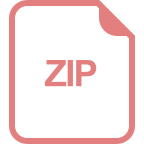
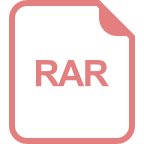
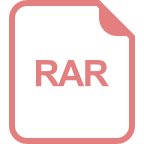
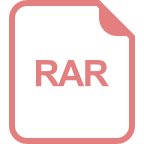
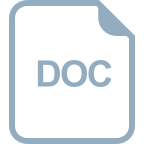
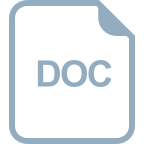
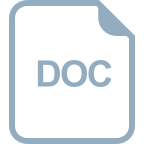
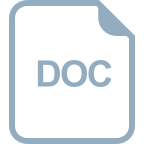