c++建立一个单向链表,将数组中的学生信息存放到链表中,并尝试再链表中进行排序。
时间: 2023-06-15 15:06:47 浏览: 57
下面是一个示例代码,实现了将学生信息存放到单向链表中并进行排序:
```c++
#include <iostream>
#include <string>
using namespace std;
struct Student {
string name;
int age;
float score;
Student* next;
Student() : name(""), age(0), score(0), next(nullptr) {}
Student(string n, int a, float s) : name(n), age(a), score(s), next(nullptr) {}
};
void addStudent(Student*& head, string name, int age, float score) {
Student* newStudent = new Student(name, age, score);
if (head == nullptr) {
head = newStudent;
} else {
Student* temp = head;
while (temp->next != nullptr) {
temp = temp->next;
}
temp->next = newStudent;
}
}
void printList(Student* head) {
Student* temp = head;
while (temp != nullptr) {
cout << temp->name << " " << temp->age << " " << temp->score << endl;
temp = temp->next;
}
}
void sortList(Student*& head) {
if (head == nullptr) {
return;
}
Student* i = head;
while (i->next != nullptr) {
Student* j = i->next;
while (j != nullptr) {
if (i->score < j->score) {
swap(i->name, j->name);
swap(i->age, j->age);
swap(i->score, j->score);
}
j = j->next;
}
i = i->next;
}
}
int main() {
Student* head = nullptr;
string name;
int age;
float score;
int n;
cout << "Enter number of students: ";
cin >> n;
for (int i = 0; i < n; i++) {
cout << "Enter name, age and score of student " << i+1 << ": ";
cin >> name >> age >> score;
addStudent(head, name, age, score);
}
cout << "Original list:" << endl;
printList(head);
sortList(head);
cout << "Sorted list:" << endl;
printList(head);
return 0;
}
```
在这个示例代码中,我们定义了一个 `Student` 结构体来存储每个学生的信息,包括姓名、年龄、分数和下一个节点的指针。然后,我们定义了三个函数:`addStudent` 用于将学生信息添加到链表中,`printList` 用于打印链表中的所有学生信息,`sortList` 用于对链表中的学生信息进行排序。
在 `main` 函数中,我们首先输入要存储的学生数量,然后通过循环将每个学生的信息添加到链表中。添加完成后,我们先打印出原始的链表,然后调用 `sortList` 函数对链表中的学生信息进行排序,最后打印出排序后的链表。
请注意,在这个示例代码中我们采用了冒泡排序算法对链表进行排序,时间复杂度为 $O(n^2)$,如果要对大量数据进行排序,建议使用更高效的排序算法。
相关推荐
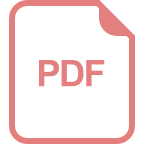
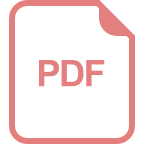
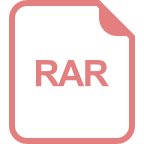














