编写C++程序,建立一个单向链表。链表结点中的数据为从键盘输入的一个字符串,但要求将该串字符按由小到大的顺序组织在链表中。
时间: 2024-02-18 17:04:29 浏览: 61
好的,这是一个很好的问题。下面是一个基于C++的单向链表的实现,可以按照要求将输入的字符串按照字典序排序后存储在链表中。
```c++
#include<iostream>
#include<string>
using namespace std;
// 定义链表结构体
struct ListNode {
string val;
ListNode* next;
ListNode() : val(""), next(NULL) {}
ListNode(string x) : val(x), next(NULL) {}
};
// 插入排序,将新节点插入到已排序的链表中
ListNode* insert(ListNode* head, ListNode* node) {
if (head == NULL) return node;
if (node->val < head->val) {
node->next = head;
return node;
}
head->next = insert(head->next, node);
return head;
}
// 读入字符串并创建链表
ListNode* createList() {
ListNode* head = NULL;
string s;
while (cin >> s) {
ListNode* node = new ListNode(s);
head = insert(head, node);
}
return head;
}
// 遍历链表并输出
void printList(ListNode* head) {
while (head != NULL) {
cout << head->val << " ";
head = head->next;
}
}
int main() {
ListNode* head = createList();
printList(head);
return 0;
}
```
在这个程序中,我们定义了一个链表结构体 `ListNode`,包含一个字符串 `val` 和一个 `next` 指针,用于连接下一个节点。我们使用 `insert` 函数将新的节点插入到已排序的链表中,从而得到一个按字典序排序的链表。最后,我们使用 `printList` 函数遍历链表并输出。
这个程序可以从标准输入中读入多个字符串并将它们按字典序排序后存储在链表中,然后输出链表中的所有字符串。
阅读全文
相关推荐



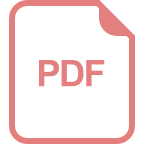
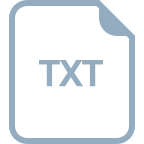
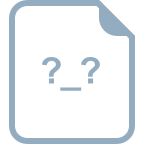
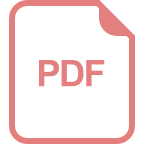
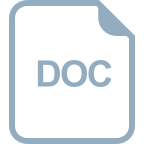
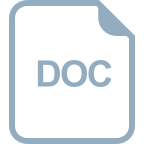
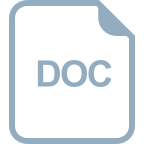
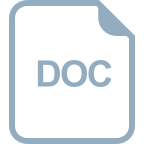
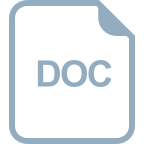
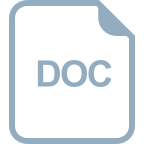
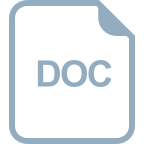
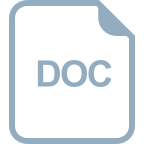
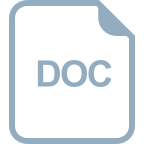