springboot项目如何在多数据源集成seata实现事务管理详细代码
时间: 2024-09-20 19:03:09 浏览: 63
Spring Boot项目集成多数据源和Seata事务管理通常需要以下几个步骤:
1. 添加依赖:首先,在你的`pom.xml`文件中添加Seata、Spring Data JPA以及Spring Cloud Transaction等依赖。
```xml
<dependencies>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-seata</artifactId>
</dependency>
<!-- 如果你打算使用JPA -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
</dependencies>
```
2. 配置数据源:在`application.yml`或`application.properties`中配置两个数据源,如`dataSource1`和`dataSource2`。
```yaml
spring:
cloud:
nacos:
discovery:
service-url: http://localhost:8848
alibaba:
seata:
registry-type: NACOS
registry-address: localhost:8647 # Seata注册中心地址
profiles:
active: multi-datasource
datasource:
# 数据源1
dataSource1:
driverClassName: com.mysql.jdbc.Driver
url: jdbc:mysql://localhost:3306/db1
username: user1
password: pass1
# 数据源2
dataSource2:
driverClassName: com.mysql.jdbc.Driver
url: jdbc:mysql://localhost:3307/db2
username: user2
password: pass2
```
3. 创建事务管理器:使用@GlobalTransactional注解,并指定对应的数据源。
```java
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.context.annotation.Configuration;
import org.springframework.transaction.annotation.EnableTransactionManagement;
@Configuration
@EnableTransactionManagement(transactionManager = "transactionManager1")
public class DataSourceConfig1 {
// 使用特定数据源的事务管理
@Autowired
@Qualifier("dataSource1")
private PlatformTransactionManager transactionManager1;
}
@Configuration
@EnableTransactionManagement(transactionManager = "transactionManager2")
public class DataSourceConfig2 {
// 类似上一步骤,这里使用的是dataSouce2
@Autowired
@Qualifier("dataSource2")
private PlatformTransactionManager transactionManager2;
}
```
4. 定义事务管理接口:为了能够跨数据源操作,创建一个公共接口,并在实际业务服务中注入这个接口。
```java
public interface TransactionalService {
void doBusiness(@Qualifier("transactionManager1") PlatformTransactionManager tm1,
@Qualifier("transactionManager2") PlatformTransactionManager tm2);
}
```
5. 在业务服务中使用事务管理接口:
```java
@Service
public class BusinessServiceImpl implements TransactionalService {
@Override
public void doBusiness(PlatformTransactionManager tm1, PlatformTransactionManager tm2) {
tm1.getTransaction();
// 对于dataSource1的操作
try {
// ...
} finally {
tm1.rollback(null); // 或者tm1.commit();取决于是否成功
}
tm2.getTransaction();
// 对于dataSource2的操作
try {
// ...
} finally {
tm2.rollback(null); // 或者tm2.commit();取决于是否成功
}
}
}
```
阅读全文
相关推荐
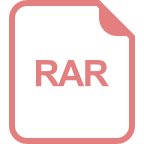
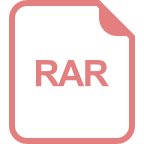
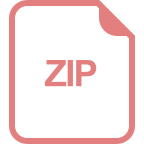





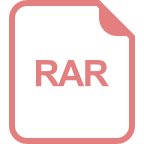
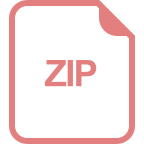
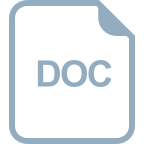
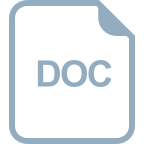






