创建一个单链表,并将最大的元素移到前边,最小的元素移到后边python
时间: 2024-09-13 17:14:56 浏览: 32
创建一个单链表并调整最大和最小元素的位置可以通过以下步骤完成:
1. 定义单链表节点类:首先我们需要定义一个单链表节点的类,它包含数据域和指向下一个节点的指针。
2. 创建单链表:然后根据给定的数据创建一个单链表。
3. 遍历链表找出最大和最小值:遍历链表,同时记录最大和最小值及其对应的节点。
4. 断开和重新链接节点:根据找到的最大和最小值,断开对应的节点,并将它们重新连接到链表的指定位置。
以下是用Python实现的代码示例:
```python
class ListNode:
def __init__(self, value=0, next=None):
self.val = value
self.next = next
def create_linked_list(lst):
if not lst:
return None
head = ListNode(lst[0])
current = head
for value in lst[1:]:
current.next = ListNode(value)
current = current.next
return head
def move_max_min(head):
if not head or not head.next:
return head
# 初始化最大最小节点和它们的前驱节点
min_node = max_node = head
prev_min = prev_max = None
current = head.next
# 初始化遍历中的第一个节点为最小值节点
current_min = min_node
while current:
if current.val < min_node.val:
min_node, prev_min = current, current
current_min = min_node
elif current.val > max_node.val:
max_node, prev_max = current, current
current = current.next
# 如果最小值已经在头节点,不需要调整
if current_min != min_node:
# 移除最大值节点
if prev_max:
prev_max.next = max_node.next
else: # 最大值节点在头节点
head = max_node.next
# 移除最小值节点
prev_min.next = min_node.next
# 将最大值节点连接到头节点,最小值节点连接到最后
min_node.next = None
max_node.next = head
head = max_node
current_min.next = min_node
return head
# 示例使用
lst = [1, 5, 3, 2, 4]
head = create_linked_list(lst)
new_head = move_max_min(head)
current = new_head
while current:
print(current.val, end=" ")
current = current.next
```
这段代码首先定义了一个链表节点类和两个函数:`create_linked_list`用于从列表创建链表,`move_max_min`用于调整链表中最大和最小值的位置。最后通过示例展示了如何使用这些函数。
相关推荐
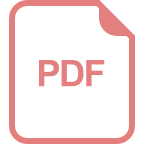
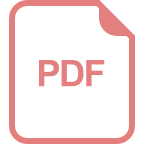














