结构体数组的冒泡paixu
时间: 2024-06-23 13:02:08 浏览: 7
在C语言中,结构体数组的冒泡排序(Bubble Sort)是一种简单的排序算法,它反复遍历数组,比较相邻元素,如果它们的顺序错误就把它们交换过来,直到整个序列变为有序。对于结构体数组,我们需要定义一个比较函数,用于根据结构体中的某个字段进行排序。
下面是结构体数组冒泡排序的一般步骤:
1. 定义一个结构体类型,包含需要排序的属性。
2. 声明一个结构体数组,并初始化它。
3. 定义一个比较函数(通常作为外部函数),接收两个结构体指针作为参数,根据其中的字段进行比较。
4. 主排序循环:
a. 对数组的所有元素执行两两比较,如果前一个元素大于后一个元素(根据比较函数),则交换它们。
b. 重复这个过程,每次减少未排序部分的长度,直到数组完全排序或已经无更多交换操作。
5. 完成排序后,结构体数组中的元素按指定字段排列有序。
相关问题
c语言结构体数组冒泡排序
以下是C语言结构体数组冒泡排序的示例代码:
```c
#include <stdio.h>
#include <string.h>
struct Student {
char name[20];
int score;
};
void bubbleSort(struct Student arr[], int n) {
int i, j;
struct Student temp;
for (i = 0; i < n - 1; i++) {
for (j = 0; j < n - i - 1; j++) {
if (arr[j].score > arr[j + 1].score) {
temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
int main() {
struct Student students[5] = {
{"Tom", 80},
{"Jerry", 70},
{"Alice", 90},
{"Bob", 85},
{"David", 75}
};
int i;
printf("Before sorting:\n");
for (i = 0; i < 5; i++) {
printf("%s: %d\n", students[i].name, students[i].score);
}
bubbleSort(students, 5);
printf("After sorting:\n");
for (i = 0; i < 5; i++) {
printf("%s: %d\n", students[i].name, students[i].score);
}
return 0;
}
```
该示例代码中定义了一个结构体`Student`,包含学生姓名和分数两个成员变量。`bubbleSort`函数实现了冒泡排序算法,其中`arr`参数为结构体数组,`n`参数为数组长度。在`main`函数中定义了一个包含5个学生信息的结构体数组`students`,并输出排序前和排序后的学生信息。
结构体实现数组冒泡排序
好的,以下是结构体实现数组冒泡排序的示例代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_SIZE 100
typedef struct {
int num;
char name[20];
int score;
} Student;
void bubbleSort(Student arr[], int n) {
int i, j;
Student temp;
for (i = 0; i < n-1; i++) {
for (j = 0; j < n-i-1; j++) {
if (arr[j].score < arr[j+1].score) {
temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
}
}
int main() {
int n, i, j;
Student students[MAX_SIZE];
printf("Enter the number of students: ");
scanf("%d", &n);
for (i = 0; i < n; i++) {
printf("\nEnter details of student %d:\n", i+1);
printf("Number: ");
scanf("%d", &students[i].num);
printf("Name: ");
scanf("%s", students[i].name);
printf("Score: ");
scanf("%d", &students[i].score);
}
bubbleSort(students, n);
printf("\nStudents sorted by descending score:\n");
for (i = 0; i < n; i++) {
printf("%d\t%s\t%d\n", students[i].num, students[i].name, students[i].score);
}
return 0;
}
```
在这个示例中,我们定义了一个结构体 `Student` 来表示每个学生的信息,包括学号、姓名和分数。然后我们定义了一个 `bubbleSort` 函数来对学生信息进行冒泡排序。最后在 `main` 函数中,我们从用户输入读取学生信息,然后调用 `bubbleSort` 函数进行排序,并输出排好序的学生信息。
需要注意的是,结构体数组的排序方式和普通的数组排序方式是一样的,唯一的区别是比较的时候需要比较结构体的某个成员。在这个示例中,我们使用学生的分数来进行比较。
相关推荐
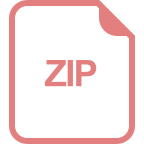
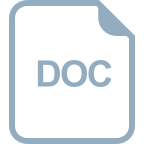
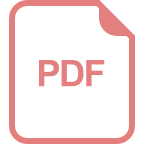












