pyqt5中setproperty的用法
时间: 2024-12-14 19:22:30 浏览: 6
在PyQt5中,`setProperty`是一个用于设置组件属性的方法,通常应用于QObjects,如QPushButton、QLineEdit等。它的基本语法如下:
```python
QObject.setProperty(name, value)
```
其中,
- `name` 参数是一个字符串,表示你要设置的属性名。比如对于一个QPushButton,常见的属性有 "text" (文本) 或 "checkable" (是否可选中) 等。
- `value` 参数是要赋予该属性的新值。
例如,如果你有一个QPushButton,并想改变其文本内容:
```python
button = QPushButton()
button.setProperty("text", "新的按钮文本")
```
或者如果你想获取某个属性的当前值:
```python
text = button.property("text")
```
注意,不是所有的属性都能直接通过`setProperty`设置,有些需要特定的方式(如信号槽机制)。此外,如果属性不在QObject系统中预定义,你需要使用`QMetaObject`来进行操作。
相关问题
pyqt5 中显示world文档
### 回答1:
要在 PyQt5 中显示 Word 文档,可以使用 QAxWidget(ActiveX 控件) 和 Microsoft Word 应用程序对象。以下是一个简单的示例代码:
```python
from PyQt5.QtWidgets import QApplication, QMainWindow, QAxWidget
import sys
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建 ActiveX 控件
self.word = QAxWidget("Word.Application", self)
self.setCentralWidget(self.word)
# 打开 Word 文档
self.word.dynamicCall("SetVisible(bool)", True)
self.word.setProperty("DisplayAlerts", False)
self.word.dynamicCall("Documents.Open(const QString&)", "path/to/your/word/document.docx")
if __name__ == "__main__":
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
在这个示例中,我们创建了一个 QMainWindow,并在中心窗口部件中添加了一个 QAxWidget。然后,我们使用 QAxWidget 创建了一个 Microsoft Word 应用程序对象,并使用该对象打开了 Word 文档。
请注意,此示例假定 Microsoft Word 已经安装在您的计算机上。如果没有安装,将无法使用该示例。
### 回答2:
在PyQt5中显示Word文档需要使用QAxWidget模块,首先需要先安装PyQt5和pypiwin32库。
安装pypiwin32库的命令是:pip install pypiwin32
然后,可以使用以下代码来完成在PyQt5中显示Word文档的工作:
```python
from PyQt5.QtWidgets import QApplication, QWidget, QVBoxLayout
from PyQt5.QAxContainer import QAxWidget
class WordViewer(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle("Word Viewer")
self.resize(800, 600)
layout = QVBoxLayout()
self.setLayout(layout)
self.word_widget = QAxWidget("Word.Application", self)
self.word_widget.setControl("word.Application")
layout.addWidget(self.word_widget)
# 打开并显示Word文档
self.word_widget.dynamicCall("SetVisible (bool Visible)", True)
self.word_widget.setControl("Documents.Open('your_word_document.docx')")
if __name__ == "__main__":
app = QApplication([])
word_viewer = WordViewer()
word_viewer.show()
app.exec()
```
在上述代码中,我们创建了一个名为WordViewer的自定义QWidget类,并将一个QAxWidget作为子组件添加到主窗口的布局中。然后,我们使用`dynamicCall()`方法调用Word的相关操作,打开并显示指定的Word文档。
请注意,这里的`your_word_document.docx`是你希望显示的Word文档的文件名,确保该文件与你的Python脚本在同一个目录下,或者使用正确的绝对路径。
希望这个回答对你有帮助!
### 回答3:
在使用PyQt5中显示Word文档,首先需要安装python-docx库,使用pip命令进行安装。安装完成后,可以通过以下步骤实现:
首先,导入所需的模块:
```
from PyQt5.QtWidgets import QApplication, QTextBrowser
from docx import Document
```
然后,创建一个PyQt5的应用程序:
```
app = QApplication([])
```
接下来,创建一个QTextBrowser对象,用于显示文档内容:
```
text_browser = QTextBrowser()
```
使用python-docx库打开Word文档并将内容读取到一个字符串中:
```
doc = Document('filename.docx') # 替换为实际的Word文档路径
content = ''
for paragraph in doc.paragraphs:
content += paragraph.text + '\n'
```
将文档内容设置到QTextBrowser对象中:
```
text_browser.setText(content)
```
最后,显示QTextBrowser对象,并运行PyQt5应用程序:
```
text_browser.show()
app.exec_()
```
这样,便可以在PyQt5应用程序中显示Word文档的内容。需要注意的是,此方法只能显示文本内容,对于Word中的图表、图片等内容可能无法完全显示。若需要更复杂的功能,可能需要使用其他第三方库或者在PyQt5中使用内嵌的Web浏览器组件进行显示。
pyqt5表格中添加按钮
在PyQt5中,可以通过在表格中添加按钮来实现特定的交互功能。下面是一个简单的示例,可以在表格中添加按钮并为其添加点击事件:
```python
from PyQt5.QtWidgets import QApplication, QMainWindow, QTableWidget, QTableWidgetItem, QPushButton
from PyQt5.QtCore import Qt
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.table = QTableWidget()
self.table.setColumnCount(3)
self.table.setRowCount(2)
for i in range(self.table.rowCount()):
for j in range(self.table.columnCount()-1):
self.table.setItem(i, j, QTableWidgetItem("Cell {0}-{1}".format(i, j)))
for row in range(self.table.rowCount()):
button = QPushButton("Button {0}".format(row))
button.setProperty("row", row)
button.clicked.connect(self.handleButtonClicked)
self.table.setCellWidget(row, self.table.columnCount()-1, button)
self.setCentralWidget(self.table)
def handleButtonClicked(self):
button = self.sender()
row = button.property("row")
print("Button {0} clicked in row {1}".format(button.text(), row))
if __name__ == "__main__":
app = QApplication([])
window = MainWindow()
window.show()
app.exec_()
```
在这个示例中,我们创建了一个包含3列和2行的表格,并在前两列中添加了文本单元格。我们然后遍历表格的每一行,并为每一行添加一个按钮。我们使用`setProperty`方法为每个按钮存储一个`row`属性,以便在按钮被点击时可以轻松地获取行号。最后,我们将按钮添加到表格中的第三列,并连接每个按钮的`clicked`信号到我们的`handleButtonClicked`方法上。
`handleButtonClicked`方法获取被点击的按钮并从中获取行号。通过这种方式,您可以轻松地在表格中实现各种交互功能。
阅读全文
相关推荐
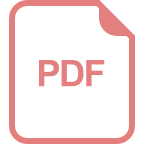
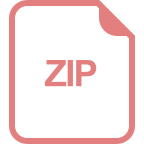
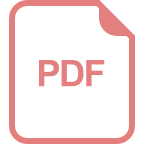













