pyqt5 QPropertyAnimation旋转动画
时间: 2024-02-05 11:04:36 浏览: 252
使用 QPropertyAnimation 实现旋转动画的步骤如下:
1. 导入必要的模块:
```python
from PyQt5.QtCore import QPropertyAnimation, QPoint, QRect, QSize, Qt
from PyQt5.QtGui import QPainter, QPen
from PyQt5.QtWidgets import QApplication, QMainWindow, QWidget
```
2. 创建一个 QWidget 子类,并实现绘制方法 `paintEvent`,在其中绘制需要旋转的图形:
```python
class Example(QWidget):
def paintEvent(self, event):
painter = QPainter(self)
painter.setRenderHint(QPainter.Antialiasing)
painter.translate(self.width() / 2, self.height() / 2)
painter.rotate(self.angle)
pen = QPen(Qt.black, 3)
painter.setPen(pen)
painter.drawRect(-50, -50, 100, 100)
```
这里绘制了一个边长为 100 的正方形,并将其绘制在窗口中心点处。
3. 创建一个 `QPropertyAnimation` 对象,并设置动画属性:
```python
self.animation = QPropertyAnimation(self, b"angle")
self.animation.setDuration(2000)
self.animation.setStartValue(0)
self.animation.setEndValue(360)
```
这里创建了一个名为 `angle` 的属性动画,并设置动画时长为 2 秒,起始值为 0,结束值为 360。
4. 启动动画:
```python
self.animation.start()
```
完整的代码如下:
```python
from PyQt5.QtCore import QPropertyAnimation, QPoint, QRect, QSize, Qt
from PyQt5.QtGui import QPainter, QPen
from PyQt5.QtWidgets import QApplication, QMainWindow, QWidget
class Example(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setGeometry(300, 300, 300, 300)
self.setWindowTitle('旋转动画')
self.show()
def paintEvent(self, event):
painter = QPainter(self)
painter.setRenderHint(QPainter.Antialiasing)
painter.translate(self.width() / 2, self.height() / 2)
painter.rotate(self.angle)
pen = QPen(Qt.black, 3)
painter.setPen(pen)
painter.drawRect(-50, -50, 100, 100)
def getAngle(self):
return self._angle
def setAngle(self, value):
self._angle = value
self.update()
angle = property(getAngle, setAngle)
if __name__ == '__main__':
app = QApplication([])
ex = Example()
# 创建动画对象
ex.animation = QPropertyAnimation(ex, b"angle")
ex.animation.setDuration(2000)
ex.animation.setStartValue(0)
ex.animation.setEndValue(360)
# 启动动画
ex.animation.start()
app.exec_()
```
运行程序后,将会看到一个旋转的正方形。
阅读全文
相关推荐
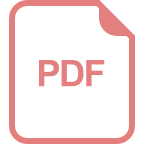
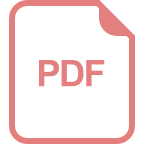
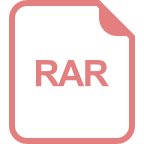




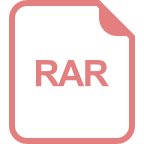
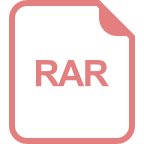
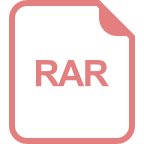
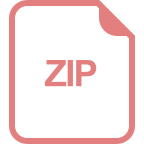
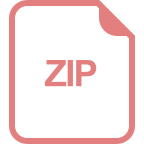
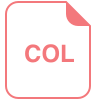
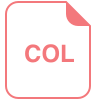
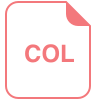



