如何用winform逐行读取txt文件中的多个关键字,并将多个关键字前后10个字符分别显示在txtbox中
时间: 2024-09-11 18:01:34 浏览: 43
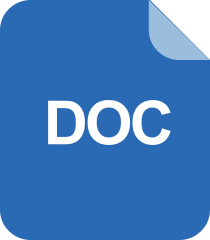
WinForm 选项卡
在WinForms应用程序中,逐行读取txt文件并显示关键字前后10个字符可以通过以下步骤实现:
1. 首先,使用`StreamReader`类来打开并逐行读取txt文件。
2. 在读取每一行时,使用字符串搜索方法(如`IndexOf`)来查找关键字。
3. 一旦找到关键字,计算关键字前后各10个字符的位置。
4. 使用`Substring`方法来获取这20个字符(10个字符在关键字前,10个字符在关键字后)。
5. 将得到的字符串设置到`TextBox`控件中显示。
下面是一个简单的示例代码:
```csharp
using System;
using System.IO;
using System.Windows.Forms;
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
string filePath = "path_to_your_file.txt"; // 指定txt文件的路径
string[] keywords = { "关键字1", "关键字2" }; // 需要查找的关键字数组
ReadFileAndDisplayKeywords(filePath, keywords);
}
private void ReadFileAndDisplayKeywords(string filePath, string[] keywords)
{
try
{
using (StreamReader reader = new StreamReader(filePath))
{
string line;
while ((line = reader.ReadLine()) != null)
{
foreach (string keyword in keywords)
{
int keywordIndex = line.IndexOf(keyword);
while (keywordIndex != -1)
{
// 获取关键字前10个字符
int start = Math.Max(0, keywordIndex - 10);
string beforeKeyword = line.Substring(start, keywordIndex - start);
// 获取关键字后10个字符
int end = Math.Min(line.Length - 1, keywordIndex + keyword.Length + 9);
string afterKeyword = line.Substring(keywordIndex + keyword.Length, end - (keywordIndex + keyword.Length));
// 在这里将beforeKeyword和afterKeyword显示到TextBox中
// 例如: textBox1.Text = beforeKeyword + afterKeyword;
keywordIndex = line.IndexOf(keyword, keywordIndex + 1); // 继续查找下一个关键字出现的位置
}
}
}
}
}
catch (Exception ex)
{
MessageBox.Show("读取文件时发生错误:" + ex.Message);
}
}
}
```
请将`path_to_your_file.txt`替换为你的实际文件路径,并根据需要调整关键字数组。
阅读全文
相关推荐
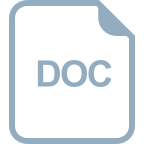
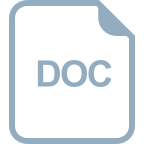







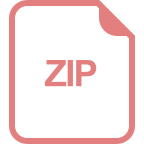
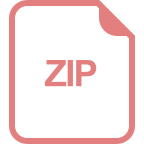
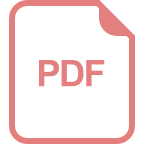
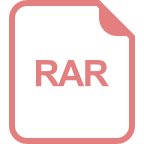
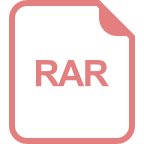
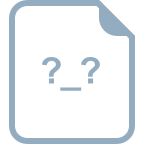
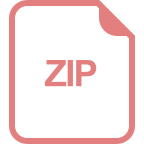
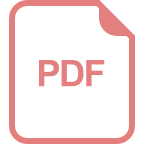
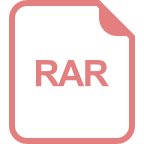