如何用winform逐行读取txt文件中的关键字,并将关键字后10个字符显示在txtbox中
时间: 2024-09-11 22:01:20 浏览: 36
在WinForms应用程序中,逐行读取文本文件中的关键字并将关键字后的10个字符显示在文本框中,可以使用以下步骤来实现:
1. 首先,你需要打开一个文本文件并逐行读取。可以使用`System.IO`命名空间中的`File.ReadAllLines`或`File.ReadLines`方法来读取文件的所有行。
2. 然后,遍历读取到的每一行字符串,使用字符串操作方法来查找关键字。
3. 如果找到关键字,截取关键字后面的10个字符(如果存在的话)。
4. 最后,将截取的字符串设置到文本框(TextBox)控件中。
以下是一个简单的示例代码:
```csharp
using System;
using System.IO;
using System.Windows.Forms;
public partial class MainForm : Form
{
public MainForm()
{
InitializeComponent();
}
private void btnReadFile_Click(object sender, EventArgs e)
{
OpenFileDialog openFileDialog = new OpenFileDialog();
if (openFileDialog.ShowDialog() == DialogResult.OK)
{
string[] lines = File.ReadAllLines(openFileDialog.FileName);
foreach (string line in lines)
{
string keyword = "关键字"; // 假设我们要找的关键字是"关键字"
int index = line.IndexOf(keyword);
if (index != -1)
{
// 确保关键字后有足够的字符
if (index + keyword.Length + 10 <= line.Length)
{
string textToShow = line.Substring(index + keyword.Length, 10);
txtResult.Text = textToShow; // 假设有一个名为txtResult的文本框控件
}
else
{
txtResult.Text = "关键字后不足10个字符";
}
break; // 如果只需要显示第一个找到的关键字后的字符,则找到后即退出循环
}
}
}
}
}
```
请注意,上述代码中的`keyword`应该替换成实际要查找的关键字,而`txtResult`是假设的文本框控件的名称,你需要根据实际情况替换成你界面上的文本框控件的名称。
阅读全文
相关推荐
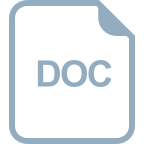
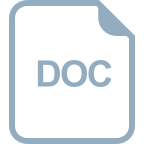
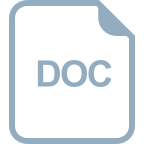







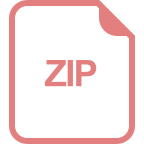
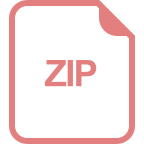
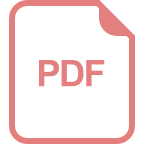
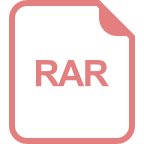
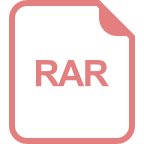
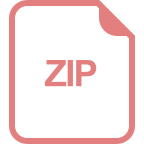
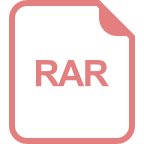
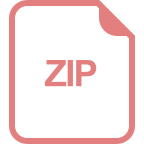
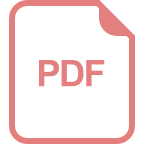