c++求一元二次方程最值
时间: 2024-10-23 22:08:12 浏览: 18
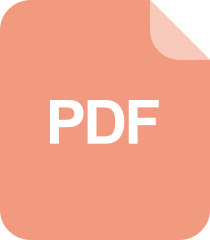
C++通过自定义函数求一元二次方程的根
在C++中,求解一元二次方程的最值通常涉及到找到该方程的根,因为二次函数在其顶点处取得最小值(如果开口向上)或最大值(如果开口向下)。如果你有一个一般形式的二次方程,例如 ax^2 + bx + c = 0,你可以使用公式法(如韦达定理或二次公式)来找出根。
首先,需要确定方程的系数a、b和c,然后计算判别式Δ = b^2 - 4ac,判断其性质:
- 当Δ > 0 时,有两个实数根,可以分别通过公式 x = [-b ± sqrt(b² - 4ac)] / (2a) 计算;
- 当Δ = 0 时,有一个重根,即x = -b / (2a),方程有唯一的实根;
- 当Δ < 0 时,有两个复数根,不适合直接计算最值。
若要寻找函数的最大值或最小值,你需要找到顶点坐标,对于标准形式y = ax^2 + bx + c,顶点的横坐标x = -b / (2a)。将这个x代入原方程即可得到对应的y值,即为函数的极值。
如果你想在程序中实现这个过程,可以创建一个类,包含方法来计算根和极值。下面是一个简单的示例:
```cpp
#include <iostream>
#include <cmath>
class QuadraticFunction {
public:
QuadraticFunction(double a, double b, double c) : a_(a), b_(b), c_(c) {}
// 计算判别式
double discriminant() const { return b_ * b_ - 4 * a_ * c_; }
// 计算两个根
std::pair<double, double> roots() const {
double discriminantSqrt = sqrt(discriminant());
if (discriminantSqrt == 0) {
return { -b_ / (2 * a_), -b_ / (2 * a_) };
} else {
return { (-b_ - discriminantSqrt) / (2 * a_), (-b_ + discriminantSqrt) / (2 * a_) };
}
}
// 计算并返回顶点坐标和极值
std::pair<double, double> vertexAndValue() const {
double x = -b_ / (2 * a_);
double y = a_ * x * x + b_ * x + c_;
return { x, y };
}
private:
double a_, b_, c_;
};
int main() {
double a, b, c;
// 输入方程系数
std::cout << "Enter coefficients for ax^2 + bx + c: ";
std::cin >> a >> b >> c;
QuadraticFunction quadratic(a, b, c);
if (quadratic.discriminant() >= 0) {
std::pair<double, double> roots = quadratic.roots();
std::pair<double, double> vertex = quadratic.vertexAndValue();
std::cout << "Roots: " << roots.first << ", " << roots.second << "\n";
std::cout << "Vertex (x, y): (" << vertex.first << ", " << vertex.second << ")\n";
std::cout << "If the function opens upward, this is the minimum value; otherwise, it's the maximum.\n";
} else {
std::cout << "The equation has complex roots and does not have a maximum or minimum within the reals.\n";
}
return 0;
}
```
阅读全文
相关推荐















