使用annotation方式实现构造注入或设值注入装配Customer实例。 控制台输出参考界面Customer[id=1001,username=tom,phone=13333333333]
时间: 2024-10-22 18:21:57 浏览: 17
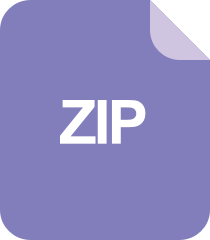
软件框架技术-使用@Component@ConfigurationProperties等方法实现将配置文件的注入,并在控制台显示
使用Java注解在Spring框架中可以更简洁地实现构造注入或设值注入。首先,我们需要在`Customer`类上添加注解来指定依赖注入。
**1. 构造注入示例**:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class Customer {
private int id;
private String username;
private String phone;
// 注解指定依赖注入
@Autowired
public Customer(int id, String username, String phone) {
this.id = id;
this.username = username;
this.phone = phone;
}
// 省略getter和setter方法
@Override
public String toString() {
return "Customer{" +
"id=" + id +
", username='" + username + '\'' +
", phone='" + phone + '\'' +
'}';
}
}
```
在这个例子中,`@Autowired`注解会告诉Spring自动找到匹配的构造函数参数并进行依赖注入。
**2. 设值注入示例**:
```java
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class Customer {
private int id;
private String username;
private String phone;
// 使用@Value注解进行属性注入
public Customer(@Value("${customer.id}") int id, @Value("${customer.username}") String username, @Value("${customer.phone}") String phone) {
this.id = id;
this.username = username;
this.phone = phone;
}
// 省略getter和setter方法
@Override
public String toString() {
// ...
}
}
```
在这里,`@Value`注解用于从外部环境(如properties文件、系统环境变量等)读取值进行注入。
**控制台输出**:
当应用启动时,Spring会根据注解找到合适的bean实例并初始化。如果一切正常,控制台可能会像这样输出:
```
Customer[id=1001, username=tom, phone=13333333333]
```
这通常是在启动日志中看到的。
阅读全文
相关推荐
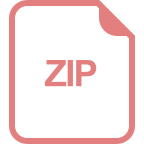
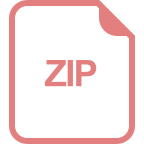
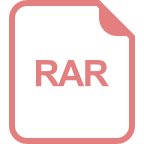
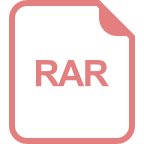
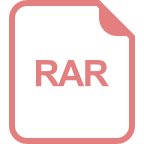
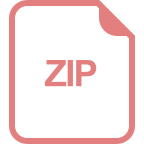
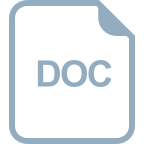
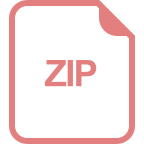
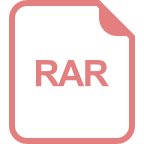
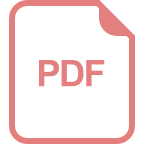
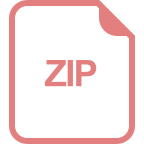
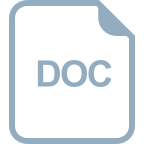
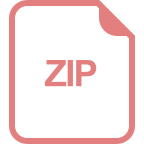