c语言add8校验和
时间: 2024-12-08 21:23:51 浏览: 8
在C语言中,校验和(如奇偶校验、CRC校验等)通常用于检查数据传输的完整性。"Add8"校验和可能是指将每个字节加上固定的8(0x8)来进行简单的一种校验,但这并不是标准的术语。例如,如果想计算一个数组中所有元素的一字节和(即ASCII值相加),你可以这样做:
```c
#include <stdio.h>
unsigned char add8_sum(const unsigned char *data, int len) {
unsigned char sum = 0;
for (int i = 0; i < len; ++i) {
sum += data[i];
}
return sum + 8; // 加上8是为了模拟简单的校验效果,实际应用可能会有其他算法
}
int main() {
const unsigned char my_array[] = {1, 2, 3, 4, 5};
unsigned char checksum = add8_sum(my_array, sizeof(my_array) / sizeof(my_array[0]));
printf("Checksum (including '8') is: %d\n", checksum);
return 0;
}
```
在这个例子中,我们遍历数组并累加每个元素的ASCII值,然后再加上固定的8。这个操作并不保证数据完整性的校验,仅作为演示用途。
相关问题
CCSDS,(8176,7156)的校验矩阵,C语言
CCSDS是Consultative Committee for Space Data Systems的缩写,是一个国际性的组织,致力于推动航天通信标准的制定和发展。CCSDS使用的是Reed-Solomon编码技术,其中(8176,7156)是一种常用的编码方式。
下面是一个使用C语言实现(8176,7156)校验矩阵的例子:
```c
#define N 8176
#define K 7156
// 生成矩阵的函数
void generate_matrix(int H[N][N-K]) {
int i, j, k, q;
int alpha[K], omega[N-K];
int p = 0x11d; // 生成多项式
// 计算alpha和omega数组
alpha[0] = 1;
for (i = 0; i < K-1; i++) {
alpha[i+1] = gf_mul(alpha[i], 2, p);
}
for (i = 0; i < N-K-1; i++) {
omega[i] = gf_pow(alpha[i], K, p);
}
// 生成矩阵
for (i = 0; i < N-K; i++) {
for (j = 0; j < N; j++) {
H[i][j] = gf_pow(alpha[j], i, p);
if (i > 0) {
H[i][j] = gf_add(H[i][j], gf_mul(omega[i-1], gf_pow(alpha[j], K-1, p), p), p);
}
}
}
}
// 有限域上的加法
int gf_add(int a, int b, int p) {
return a ^ b;
}
// 有限域上的乘法
int gf_mul(int a, int b, int p) {
int c = 0;
while (b > 0) {
if (b & 1) {
c ^= a;
}
a <<= 1;
if (a & (1 << K)) {
a ^= p;
}
b >>= 1;
}
return c;
}
// 有限域上的指数运算
int gf_pow(int a, int b, int p) {
int c = 1;
while (b > 0) {
if (b & 1) {
c = gf_mul(c, a, p);
}
a = gf_mul(a, a, p);
b >>= 1;
}
return c;
}
```
这里使用了有限域上的加法、乘法和指数运算来生成矩阵。需要注意的是,有限域上的除法并不是简单的数学除法,需要使用扩展欧几里得算法来实现。
用c语言表示
以下是使用 C 语言实现的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_EMPLOYEE_NUM 1000
struct Employee {
char id[10];
char name[50];
float basic_salary;
float position_salary;
float allowance;
float medical_insurance;
float accumulation_fund;
float total_salary;
};
struct Employee employees[MAX_EMPLOYEE_NUM];
int employee_count = 0;
void add_employee() {
struct Employee e;
printf("Enter employee ID: ");
scanf("%s", e.id);
printf("Enter employee name: ");
scanf("%s", e.name);
printf("Enter basic salary: ");
scanf("%f", &e.basic_salary);
printf("Enter position salary: ");
scanf("%f", &e.position_salary);
printf("Enter allowance: ");
scanf("%f", &e.allowance);
printf("Enter medical insurance: ");
scanf("%f", &e.medical_insurance);
printf("Enter accumulation fund: ");
scanf("%f", &e.accumulation_fund);
e.total_salary = e.basic_salary + e.position_salary + e.allowance
- e.medical_insurance - e.accumulation_fund;
employees[employee_count++] = e;
printf("Employee added successfully.\n");
}
void display_all_employee() {
printf("%-5s %-10s %-10s %-10s %-10s %-10s %-10s %-10s\n",
"No.", "ID", "Name", "Basic", "Position", "Allowance", "Medical", "Fund");
for (int i = 0; i < employee_count; i++) {
printf("%-5d %-10s %-10s %-10.2f %-10.2f %-10.2f %-10.2f %-10.2f\n",
i+1, employees[i].id, employees[i].name,
employees[i].basic_salary, employees[i].position_salary, employees[i].allowance,
employees[i].medical_insurance, employees[i].accumulation_fund);
}
}
void display_employee() {
char id[10];
printf("Enter employee ID: ");
scanf("%s", id);
for (int i = 0; i < employee_count; i++) {
if (strcmp(employees[i].id, id) == 0) {
printf("%-10s %-10s %-10s %-10s %-10s %-10s %-10s %-10s\n",
"ID", "Name", "Basic", "Position", "Allowance", "Medical", "Fund", "Total");
printf("%-10s %-10s %-10.2f %-10.2f %-10.2f %-10.2f %-10.2f %-10.2f\n",
employees[i].id, employees[i].name,
employees[i].basic_salary, employees[i].position_salary, employees[i].allowance,
employees[i].medical_insurance, employees[i].accumulation_fund,
employees[i].total_salary);
return;
}
}
printf("Employee not found.\n");
}
void edit_employee() {
char id[10];
printf("Enter employee ID: ");
scanf("%s", id);
for (int i = 0; i < employee_count; i++) {
if (strcmp(employees[i].id, id) == 0) {
printf("Enter new basic salary: ");
scanf("%f", &employees[i].basic_salary);
printf("Enter new position salary: ");
scanf("%f", &employees[i].position_salary);
printf("Enter new allowance: ");
scanf("%f", &employees[i].allowance);
printf("Enter new medical insurance: ");
scanf("%f", &employees[i].medical_insurance);
printf("Enter new accumulation fund: ");
scanf("%f", &employees[i].accumulation_fund);
employees[i].total_salary = employees[i].basic_salary + employees[i].position_salary
+ employees[i].allowance - employees[i].medical_insurance - employees[i].accumulation_fund;
printf("Employee information updated.\n");
return;
}
}
printf("Employee not found.\n");
}
void delete_employee() {
char id[10];
printf("Enter employee ID: ");
scanf("%s", id);
for (int i = 0; i < employee_count; i++) {
if (strcmp(employees[i].id, id) == 0) {
for (int j = i; j < employee_count-1; j++) {
employees[j] = employees[j+1];
}
employee_count--;
printf("Employee deleted successfully.\n");
return;
}
}
printf("Employee not found.\n");
}
void calculate_average_salary() {
float total_salary = 0.0;
for (int i = 0; i < employee_count; i++) {
total_salary += employees[i].total_salary;
}
printf("Average salary: %.2f\n", total_salary / employee_count);
}
void calculate_total_salary() {
float total_salary = 0.0;
for (int i = 0; i < employee_count; i++) {
total_salary += employees[i].total_salary;
}
printf("Total salary: %.2f\n", total_salary);
}
void display_employee_info() {
char id[10];
printf("Enter employee ID: ");
scanf("%s", id);
for (int i = 0; i < employee_count; i++) {
if (strcmp(employees[i].id, id) == 0) {
printf("ID: %s\n", employees[i].id);
printf("Name: %s\n", employees[i].name);
printf("Basic salary: %.2f\n", employees[i].basic_salary);
printf("Position salary: %.2f\n", employees[i].position_salary);
printf("Allowance: %.2f\n", employees[i].allowance);
printf("Medical insurance: %.2f\n", employees[i].medical_insurance);
printf("Accumulation fund: %.2f\n", employees[i].accumulation_fund);
printf("Total salary: %.2f\n", employees[i].total_salary);
return;
}
}
printf("Employee not found.\n");
}
int main() {
int choice;
while (1) {
printf("\n");
printf("1. Add employee\n");
printf("2. Display all employees\n");
printf("3. Display employee information\n");
printf("4. Edit employee information\n");
printf("5. Delete employee\n");
printf("6. Calculate average salary\n");
printf("7. Calculate total salary\n");
printf("8. Display employee's own information\n");
printf("9. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_employee();
break;
case 2:
display_all_employee();
break;
case 3:
display_employee();
break;
case 4:
edit_employee();
break;
case 5:
delete_employee();
break;
case 6:
calculate_average_salary();
break;
case 7:
calculate_total_salary();
break;
case 8:
display_employee_info();
break;
case 9:
exit(0);
default:
printf("Invalid choice.\n");
}
}
return 0;
}
```
注意,这只是一个简单的示例,实际应用中可能需要更加完善的错误处理逻辑和数据校验。
阅读全文
相关推荐
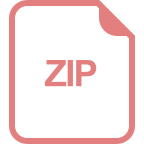
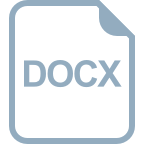
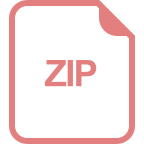
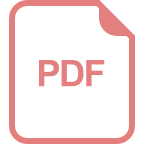
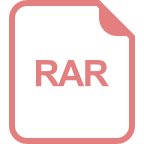
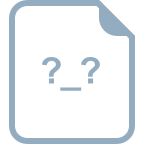
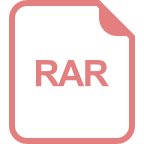
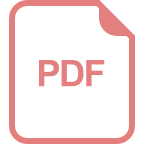
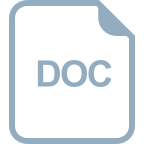
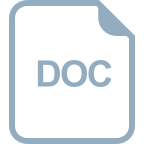
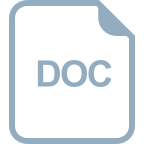
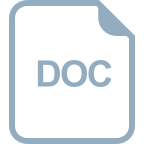
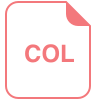
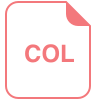
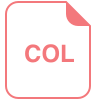
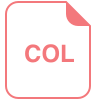
