用read方式如何读取can socket的硬件时间戳
时间: 2024-09-07 17:04:27 浏览: 154
在使用CAN socket进行通信时,通常你会通过socket发送和接收CAN帧。大多数操作系统和CAN硬件驱动程序都支持在CAN帧中包含一个硬件时间戳,该时间戳是在帧到达CAN接口时由硬件自动添加的。使用read方式读取CAN帧时,可以通过相应的API来获取这个时间戳。
以Linux下的socketCAN为例,你可以使用`read`函数从CAN socket读取数据。这个操作会返回一个`帧结构体`(`can_frame`),该结构体包含了`can_id`、`can_dlc`、数据字段以及一个`canTiming`结构体。`canTiming`结构体包含了时间戳的详细信息。
读取硬件时间戳的具体方法依赖于你使用的编程语言和CAN设备驱动程序。在C语言中,读取CAN帧通常像这样:
```c
struct can_frame frame;
int nbytes = read(can_socket, &frame, sizeof(struct can_frame));
```
如果硬件时间戳可用,你可以从`frame.canTiming`中获取到时间戳信息。以下是在Linux系统中使用socketCAN接口获取时间戳的一个示例代码段:
```c
#include <stdio.h>
#include <string.h>
#include <unistd.h>
#include <net/if.h>
#include <sys/ioctl.h>
#include <sys/socket.h>
#include <sys/types.h>
#include <linux/can.h>
#include <linux/can/raw.h>
int main()
{
int s;
int nbytes;
struct sockaddr_can addr;
struct ifreq ifr;
struct can_frame frame;
struct canfd_frame canfd_frame;
// 创建一个socket
s = socket(PF_CAN, SOCK_RAW, CAN_RAW);
// 获取接口名称
strcpy(ifr.ifr_name, "can0");
// 获取接口索引
ioctl(s, SIOCGIFINDEX, &ifr);
// 创建socketCAN的地址
addr.can_family = AF_CAN;
addr.can_ifindex = ifr.ifr_ifindex;
// 绑定socket到地址
bind(s, (struct sockaddr *)&addr, sizeof(addr));
// 读取数据
nbytes = read(s, &canfd_frame, sizeof(canfd_frame));
if (nbytes < 0) {
perror("canfd_frame read");
return 1;
}
// 输出时间戳
printf("timestamp = %u\n", canfd_frame.can_id & CAN_ERR_MASK);
close(s);
return 0;
}
```
上面的代码片段中,`can_id`字段的高8位包含了时间戳信息。时间戳通常表示为从某个参考点开始的微秒数。
请注意,具体的实现可能会因为不同的CAN控制器、驱动程序或操作系统版本而有所不同,所以你可能需要查阅特定硬件或软件的开发文档来找到最适合你的实现方法。
阅读全文
相关推荐
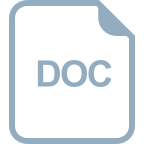
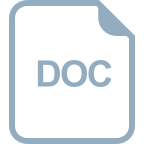
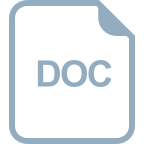
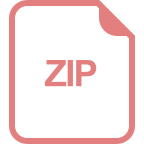
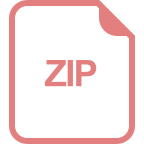
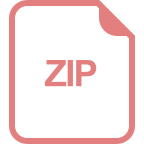
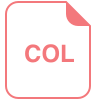
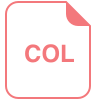
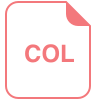
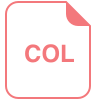
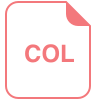
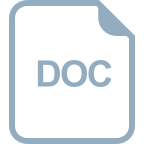
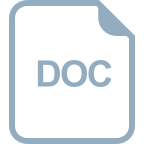
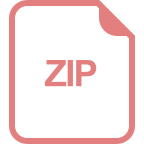
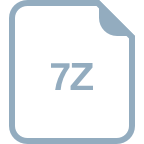
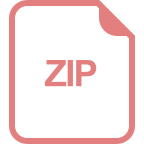
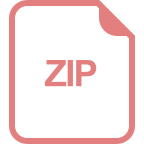
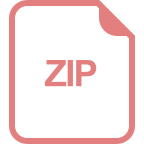