输入一个年份,一个月份(使用一个 BufferedReader),判断该年该月有多少天(使用switch,注意要判断2月是多少天)java
时间: 2024-09-22 20:06:08 浏览: 35
在Java中,你可以编写一个简单的程序来实现这个功能。这里是一个示例代码片段,它会通过`BufferedReader`读取用户输入的年份和月份,然后使用`switch`语句计算每个月的天数:
```java
import java.io.BufferedReader;
import java.io.IOException;
public class MonthDays {
public static void main(String[] args) {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
System.out.println("请输入年份:");
String yearStr = reader.readLine();
int year = Integer.parseInt(yearStr);
System.out.println("请输入月份(1-12):");
String monthStr = reader.readLine();
int month = Integer.parseInt(monthStr);
int daysInMonth = 0;
switch (month) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
daysInMonth = 31;
break;
case 4:
case 6:
case 9:
case 11:
daysInMonth = 30;
break;
// 特殊情况:闰年的二月有29天
case 2:
if (isLeapYear(year)) {
daysInMonth = 29;
} else {
daysInMonth = 28;
}
break;
default:
System.out.println("无效的月份");
return; // 结束程序
}
System.out.printf("年份 %d 的 %d 月有 %d 天.\n", year, month, daysInMonth);
try {
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
// 判断是否是闰年
private static boolean isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
}
```
在这个程序中,我们首先检查输入的月份,并根据月份确定天数。对于二月,我们额外检查了是否为闰年。最后,我们关闭`BufferedReader`以释放资源。
阅读全文
相关推荐
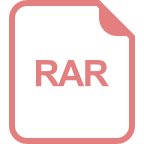
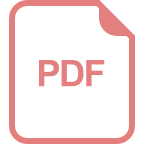
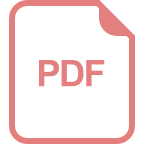
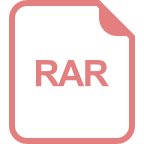
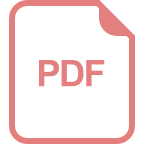
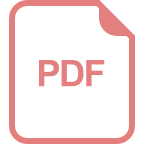
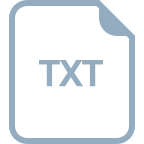
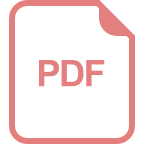
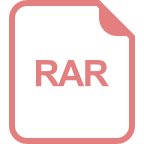
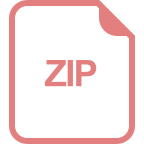
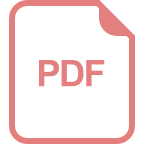
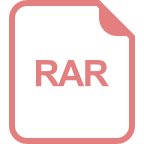
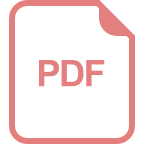
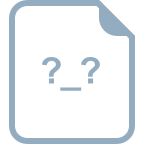
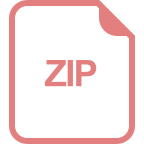
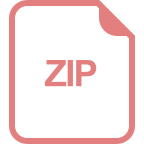
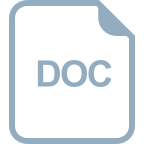