如何用C语言编写一个程序,它能接受用户输入的日期并输出对应的星期几?
时间: 2024-11-10 13:26:42 浏览: 12
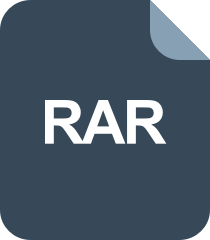
C语言: 输入任意年月日,求该天是星期几

在C语言中,我们可以使用标准库中的time.h和strftime函数来实现这个功能。以下是一个简单的示例程序,它会接收用户的日期输入(格式如"YYYY-MM-DD"),然后输出对应的英文星期:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define WEEKDAYS "Sunday Monday Tuesday Wednesday Thursday Friday Saturday"
int main() {
char input_date[10];
time_t timestamp;
struct tm *date;
printf("Enter a date in format YYYY-MM-DD: ");
scanf("%s", input_date);
// Convert string to time_t (Unix timestamp)
if (strptime(input_date, "%Y-%m-%d", &date) == NULL) {
perror("Error parsing date");
return 1;
}
// Get the current timestamp and set it to the parsed date
timestamp = mktime(date);
// Use strftime to format the timestamp as a string with day of week
char formatted_date[8];
strftime(formatted_date, sizeof(formatted_date), "%A", date);
printf("The date %s is %s.\n", input_date, formatted_date);
return 0;
}
```
在这个程序中,`strptime`函数用于解析日期字符串,`mktime`将结构体tm转换为时间戳,最后`strftime`函数格式化输出为包含星期的字符串。
阅读全文
相关推荐
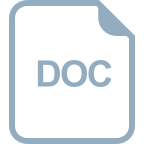
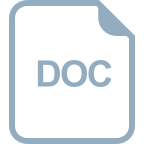

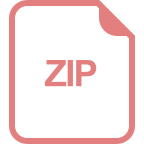






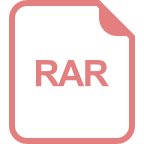
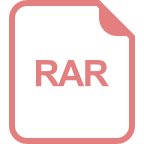
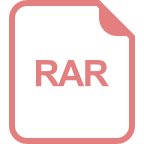
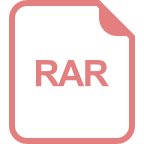
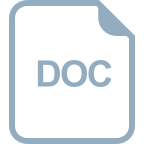


