以此文件编写信息系统代码
时间: 2024-11-25 09:13:43 浏览: 4
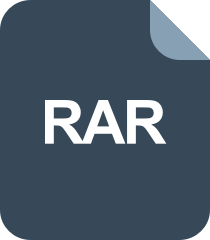
易语言源码取系统启动信息.rar
根据提供的《系统分析报告.doc》,以下是使用Python和SQLite编写的宠物体重管理系统的简化版本代码示例。此示例实现了用户注册、登录、添加宠物、记录体重和查询体重历史等功能。
### 环境准备
首先,确保安装了 `sqlite3` 模块(通常 Python 自带)。
### 数据库初始化
```python
import sqlite3
from datetime import datetime, timedelta
def init_db():
conn = sqlite3.connect('pet_weight.db')
c = conn.cursor()
# 创建用户表
c.execute('''CREATE TABLE IF NOT EXISTS users (
id INTEGER PRIMARY KEY,
username TEXT UNIQUE,
password TEXT
)''')
# 创建宠物表
c.execute('''CREATE TABLE IF NOT EXISTS pets (
id INTEGER PRIMARY KEY,
user_id INTEGER,
pet_name TEXT,
FOREIGN KEY(user_id) REFERENCES users(id)
)''')
# 创建宠物体重记录表
c.execute('''CREATE TABLE IF NOT EXISTS pet_weights (
id INTEGER PRIMARY KEY,
pet_id INTEGER,
weight REAL,
recorded_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
FOREIGN KEY(pet_id) REFERENCES pets(id)
)''')
conn.commit()
conn.close()
init_db()
```
### 用户注册
```python
def register_user(username, password):
conn = sqlite3.connect('pet_weight.db')
c = conn.cursor()
try:
c.execute("INSERT INTO users (username, password) VALUES (?, ?)", (username, password))
conn.commit()
print(f"User {username} registered successfully.")
except sqlite3.IntegrityError:
print(f"Username {username} already exists.")
conn.close()
```
### 用户登录
```python
def login_user(username, password):
conn = sqlite3.connect('pet_weight.db')
c = conn.cursor()
c.execute("SELECT * FROM users WHERE username=? AND password=?", (username, password))
user = c.fetchone()
if user:
print(f"Welcome, {username}!")
return True
else:
print("Invalid username or password.")
return False
conn.close()
```
### 添加宠物
```python
def add_pet(user_id, pet_name):
conn = sqlite3.connect('pet_weight.db')
c = conn.cursor()
c.execute("INSERT INTO pets (user_id, pet_name) VALUES (?, ?)", (user_id, pet_name))
conn.commit()
print(f"Pet {pet_name} added successfully.")
conn.close()
```
### 记录宠物体重
```python
def record_weight(pet_id, weight):
conn = sqlite3.connect('pet_weight.db')
c = conn.cursor()
c.execute("INSERT INTO pet_weights (pet_id, weight) VALUES (?, ?)", (pet_id, weight))
conn.commit()
print(f"Weight for pet ID {pet_id} recorded successfully.")
conn.close()
```
### 查询宠物体重历史
```python
def get_weight_history(pet_id):
conn = sqlite3.connect('pet_weight.db')
c = conn.cursor()
c.execute("SELECT weight, recorded_at FROM pet_weights WHERE pet_id=? ORDER BY recorded_at", (pet_id,))
history = c.fetchall()
if history:
print(f"Weight history for pet ID {pet_id}:")
for weight, recorded_at in history:
print(f"Weight: {weight}, Recorded at: {recorded_at}")
else:
print(f"No weight records found for pet ID {pet_id}.")
conn.close()
```
### 主程序
```python
def main():
while True:
print("\n1. Register\n2. Login\n3. Add Pet\n4. Record Weight\n5. Get Weight History\n6. Exit")
choice = input("Choose an option: ")
if choice == '1':
username = input("Enter username: ")
password = input("Enter password: ")
register_user(username, password)
elif choice == '2':
username = input("Enter username: ")
password = input("Enter password: ")
if login_user(username, password):
user_id = get_user_id(username)
print(f"Logged in as user ID: {user_id}")
elif choice == '3':
user_id = int(input("Enter your user ID: "))
pet_name = input("Enter pet name: ")
add_pet(user_id, pet_name)
elif choice == '4':
pet_id = int(input("Enter pet ID: "))
weight = float(input("Enter weight: "))
record_weight(pet_id, weight)
elif choice == '5':
pet_id = int(input("Enter pet ID: "))
get_weight_history(pet_id)
elif choice == '6':
break
else:
print("Invalid choice. Please try again.")
if __name__ == "__main__":
main()
```
### 辅助函数
```python
def get_user_id(username):
conn = sqlite3.connect('pet_weight.db')
c = conn.cursor()
c.execute("SELECT id FROM users WHERE username=?", (username,))
user_id = c.fetchone()[0]
conn.close()
return user_id
```
这个示例代码涵盖了系统的基本功能,并且可以根据需要进一步扩展和完善。希望这对你有帮助!
阅读全文
相关推荐
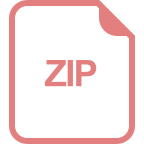
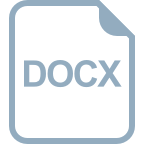












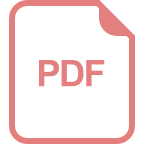
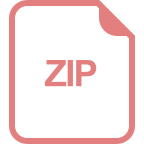
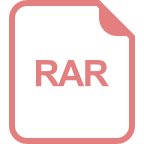
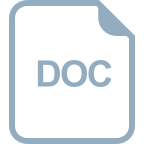