如何在WPF中创建一个带有分组的下拉菜单?
时间: 2024-09-10 18:19:57 浏览: 19
在WPF中创建一个带有分组的下拉菜单可以通过使用`ComboBox`控件来实现。这通常是通过将`ComboBox`的`ItemsSource`属性绑定到一个分组的集合来完成的,比如使用`ObservableCollection`,并且这个集合的每个元素都是一个实现了`INotifyPropertyChanged`接口的对象,以便在运行时动态地更改数据。
以下是一个简单的示例代码,展示了如何在WPF中创建带有分组的下拉菜单:
```xml
<Window x:Class="WpfApp.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="带有分组的下拉菜单" Height="200" Width="300">
<Grid>
<ComboBox ItemsSource="{Binding Items}">
<ComboBox.ItemTemplate>
<DataTemplate>
<HierarchicalDataTemplate ItemsSource="{Binding SubItems}">
<TextBlock Text="{Binding Name}"/>
</HierarchicalDataTemplate>
</DataTemplate>
</ComboBox.ItemTemplate>
</ComboBox>
</Grid>
</Window>
```
在代码后面,你需要设置相应的数据模型,例如:
```csharp
public class Item
{
public string Name { get; set; }
public ObservableCollection<SubItem> SubItems { get; set; }
}
public class SubItem
{
public string Name { get; set; }
}
public partial class MainWindow : Window
{
public ObservableCollection<Item> Items { get; set; }
public MainWindow()
{
InitializeComponent();
this.DataContext = this;
Items = new ObservableCollection<Item>();
// 添加分组数据
Items.Add(new Item
{
Name = "分组1",
SubItems = new ObservableCollection<SubItem>
{
new SubItem { Name = "子项1.1" },
new SubItem { Name = "子项1.2" }
}
});
Items.Add(new Item
{
Name = "分组2",
SubItems = new ObservableCollection<SubItem>
{
new SubItem { Name = "子项2.1" },
new SubItem { Name = "子项2.2" }
}
});
}
}
```
在这个例子中,`Item` 类代表了分组,而`SubItem` 类代表了属于每个分组的子项。`MainWindow`构造函数中,我们初始化了一些分组和子项数据,并将它们添加到`Items`集合中。`ComboBox`的`ItemTemplate`使用了`HierarchicalDataTemplate`来展示每个分组的名称,以及它的子项。
相关推荐
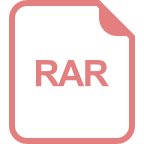
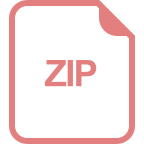
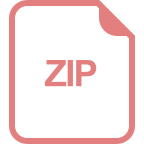














