编程实现 导弹飞行动力学模型 输出一条弹道
时间: 2024-09-28 16:14:42 浏览: 26
导弹飞行动力学模型通常涉及牛顿运动定律、空气阻力模型和重力影响。在Python中,我们可以使用科学计算库如`scipy`来简化计算过程。下面是一个简化的弹道模型实现,假设子弹以一定的初速度和角度发射:
```python
import math
from scipy.integrate import solve_ivp
from scipy.optimize import fsolve
# 定义物理常量
g = 9.81 # 重力加速度
rho = 1.225 # 空气密度
A = 0.1 # 弹丸横截面积
Cd = 0.47 # 空气阻力系数
m = 0.1 # 弹丸质量
# 初始条件
v0 = 1000 # 初速 (m/s)
theta = math.radians(45) # 发射角 (度)
# 动力学方程(忽略滚转和俯仰)
def projectile_equations(t, y):
x, v_x, vy = y
a_x = -g * math.sin(theta)
a_y = -g * math.cos(theta) - (0.5 * rho * A * Cd * v_x**2) / m
return [v_x, a_x, a_y]
# 解决微分方程
t_span = (0, 10) # 时间范围 (秒),直到10秒后停止
y0 = [0, v0 * math.cos(theta), v0 * math.sin(theta)] # 初始位置和速度
solution = solve_ivp(projectile_equations, t_span, y0)
# 输出轨迹数据
x = solution.y[0]
y = solution.y[1]
print(f"弹道轨迹:({x[-1]:.2f}, {y[-1]:.2f})在时间{solution.t[-1]:.2f}秒后落地.")
# 直接求解落点坐标(如果需要精确到某个高度)
height = 0.5 * g * solution.t[-1]**2 # 基于自由落体的高度公式
h0 = height * math.cos(math.radians(45))
vx0 = fsolve(lambda vx: projectile_equations(solution.t[-1], [solution.y[0][-1], vx, h0])[1], v0)[0]
落地时间 = fsolve(lambda t: projectile_equations(t, [solution.y[0][-1], vx0, h0])[2], solution.t[-1])[0]
print(f"若要求在特定高度{height:.2f}米处落地,预计落在 ({solution.y[0][-1] + vx0 *落地时间:.2f}, {h0:.2f})")
阅读全文
相关推荐
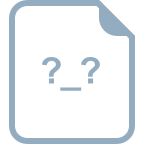
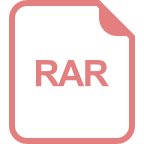
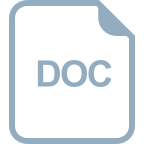
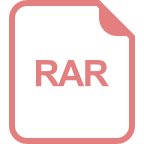
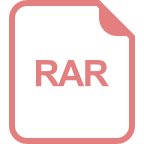
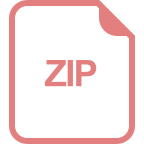
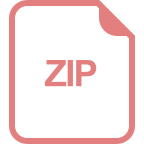
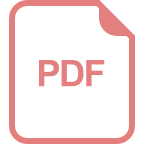
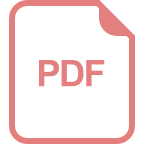
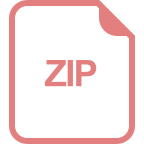
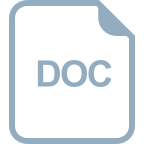