values python
时间: 2024-10-11 21:10:00 浏览: 30
在Python中,"values"这个词可以有多重含义,取决于上下文。如果是指在编程中,它可能指代以下几个意思:
1. **字典(Dictionary)中的值**:在Python的内置数据结构`dict`(字典)中,每个键(key)关联一个值(value),你可以通过键获取对应的值。例如:
```python
my_dict = {'name': 'Alice', 'age': 25}
value = my_dict['name'] # 输出:'Alice'
```
2. **函数返回值**:函数通常会有一个或多个返回值,它们表示函数执行的结果。例如:
```python
def get_value():
return 42
value = get_value() # value 的值现在是 42
```
3. **列表、元组等序列中的元素**:在这些可迭代类型中,每个位置的元素也可以称为value。如:
```python
values_list = [1, 2, 3]
first_value = values_list[0] # 输出:1
```
4. **枚举(Enum)**:Python有内置模块`enum`支持创建枚举类型,其中成员也有values属性。
**相关问题--:**
1. Python中如何访问字典的值?
2. Python函数的默认值和返回值有何区别?
3. 如何在列表和元组中设置值并访问特定索引的值?
相关问题
Python+values
`values()`是Python字典中的一个内置方法,用于获取字典中所有的值。它返回一个视图对象,其中包含字典中所有的值作为一个列表。以下是一个例子:
```python
# 创建一个字典
my_dict = {'a': 1, 'b': 2, 'c': 3}
# 使用values()方法获取所有的值
values = my_dict.values()
# 输出所有的值
print(values) # 输出:dict_values([1, 2, 3])
```
在上面的例子中,我们首先创建了一个字典`my_dict`,然后使用`values()`方法获取了所有的值,并将其存储在变量`values`中。最后,我们输出了所有的值。
how to extract common values using python
To extract common values between two lists in Python, you can use the `set` data structure and the `intersection` method. Here's an example:
```python
list1 = [1, 2, 3, 4]
list2 = [3, 4, 5, 6]
common_values = set(list1).intersection(list2)
print(common_values)
```
Output:
```
{3, 4}
```
In the above code, we convert `list1` and `list2` into sets using the `set()` function. Then, we use the `intersection()` method to find the common values between the two sets. The result is a new set `common_values` that contains the common elements.
If you want to extract common values between two columns in a pandas DataFrame, you can use the `intersect1d` function from the numpy library. Here's an example:
```python
import numpy as np
import pandas as pd
# Create an example DataFrame
df = pd.DataFrame({'A': [1, 2, 3, 4], 'B': [3, 4, 5, 6]})
# Extract common values between two columns
common_values = np.intersect1d(df['A'], df['B'])
print(common_values)
```
Output:
```
[3 4]
```
In this example, we use the `intersect1d()` function from numpy to find the common values between columns `'A'` and `'B'` in the DataFrame `df`. The result is a numpy array `common_values` containing the common elements.
阅读全文
相关推荐
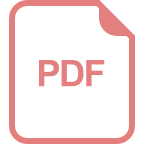
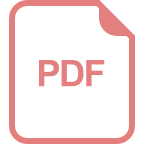
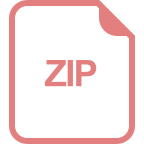













