c语言 科学计数法转换成数字
时间: 2024-12-12 16:23:09 浏览: 12
在C语言中,科学计数法通常是指将一个十进制数值表示为一个基数乘以10的幂的形式,例如1.2e3(代表1.2乘以10的3次方)。如果你想将这种字符串形式的科学计数法转换为实际的数字,可以使用`strtol`函数配合`atof`函数或者直接使用`strtod`函数。
以下是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
double scientific_to_double(const char *str) {
double num = atof(str);
// 检查是否有效
if (num == -1 && errno == ERANGE) {
fprintf(stderr, "Invalid number in scientific notation.\n");
return 0;
}
return num;
}
int main() {
const char *sci_str = "1.2e3";
double result = scientific_to_double(sci_str);
printf("The number is: %.2f\n", result);
return 0;
}
```
在这个例子中,`atof`函数用于从字符串转换到浮点数,如果输入不是有效的科学计数法,它会返回-1,并设置`errno`标志。你可以检查这个标志判断转换是否成功。
相关问题
c语言科学计数法转成数字
在C语言中,科学计数法通常是指以'e'或'E'作为指数表示的小数形式,如"1.23e4"代表1.23乘以10的4次方。要把这种字符串形式的科学计数法转换为数字,你可以使用`atof()`函数结合指数计算来完成。下面是一个简单的示例:
```c
#include <stdio.h>
#include <math.h>
double scientific_to_number(const char* str) {
long double value = atof(str);
int exponent = strcspn(str, "eE") - 1; // 找到'e'或'E'前的数字位数
if (str[strlen(str) - 1] == 'e' || str[strlen(str) - 1] == 'E') {
return pow(10, exponent + (value % 1 != 0 ? 1 : 0)) * value; // 考虑小数部分的情况
} else {
return value;
}
}
int main() {
char str[] = "1.23e4";
double num = scientific_to_number(str);
printf("Number in decimal form: %.2f\n", num);
return 0;
}
```
在这个例子中,`scientific_to_number()`函数首先通过`atof()`获取整数值,然后根据'e'或'E'的位置确定指数。如果小数点后有非零值,则考虑将指数加一。
c语言科学计数法保留有效数字
在C语言中,科学计数法(也称为指数表示法或e-notation)是一种数值表示方法,它将一个数字表示为基数(通常是10)乘以一个幂。当你需要表示非常大或非常小的数字,并希望保持精度的同时简化显示时,可以使用这种格式。
例如,如果你想保留两位有效数字,你可以这样转换和格式化一个数:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
double to_scientific_notation(double number, int precision) {
// 计算指数部分
int exponent = (int)log10(fabs(number));
// 计算调整后的基数
double adjusted_number = number / pow(10, exponent);
// 指数四舍五入到指定精度
char buffer[5]; // 足够存放整数部分和一个小数点
snprintf(buffer, sizeof(buffer), "%.*d", precision, exponent);
char* end = buffer + precision;
// 将整数部分与小数点拼接,并添加'e'或'E'
*end++ = '.';
while (*end != '\0') {
*end++ = '0'; // 预设足够多的零
}
*end++ = 'e';
if (number >= 0) {
*end++ = '+';
} else {
*end++ = '-';
}
// 添加指数
*end = '\0';
return atof(buffer); // 将字符串转换回double类型
}
int main() {
double num = 1234567890.12345;
double scientific_num = to_scientific_notation(num, 2);
printf("Original: %.2f\n", num);
printf("Scientific notation: %g\n", scientific_num);
return 0;
}
```
在这个示例中,`to_scientific_notation`函数接受一个双精度数和所需的精度,然后返回一个以科学计数法表示并保留指定有效数字的结果。
阅读全文
相关推荐
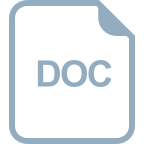
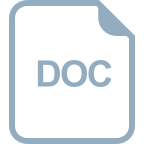
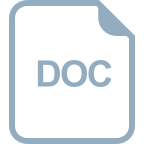
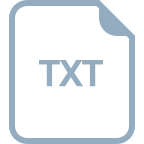












