如何用C语言实现将科学计数法转换为十进制数表示的过程?
时间: 2024-12-04 10:21:35 浏览: 20
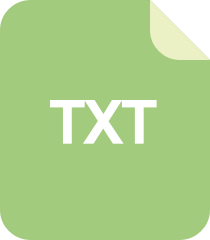
十进制数转换为二进制数.txt
在C语言中,将科学计数法转换为十进制数可以分为两个步骤:首先解析字符串,然后计算实际数值。这里是一个简单的示例,假设输入的科学计数法字符串格式正确,只包含数字和小数点:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
double sci_to_decimal(char *sci_num) {
int negative = (sci_num[0] == '-') ? 1 : 0;
char* end;
long long int power = 0;
double result = strtod(sci_num + negative, &end); // 解析数字部分
if (*end != 'e' && *end != 'E') { // 检查是否有指数
return negative ? -result : result;
}
// 获取指数部分
int exp = 0;
for (int i = 1; i < strlen(sci_num) - 1; i++) { // 跳过符号和'e'
exp += (sci_num[i] - '0');
}
if (sci_num[strlen(sci_num) - 1] == 'e' || sci_num[strlen(sci_num) - 1] == 'E')
exp -= 1; // 负指数从'e'后的字符开始计数
// 根据指数调整结果
result *= pow(10, exp);
return negative ? -result : result;
}
int main() {
char sci_input[] = "1.23e4"; // 示例输入
double decimal_result = sci_to_decimal(sci_input);
printf("Scientific notation %s converted to decimal is %.2f\n", sci_input, decimal_result);
return 0;
}
```
阅读全文
相关推荐
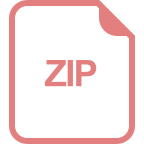
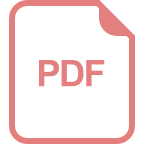
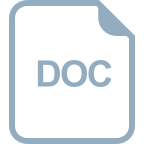
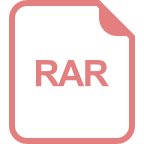
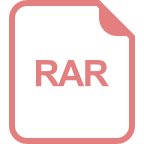
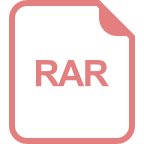
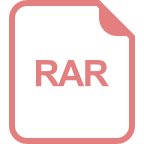
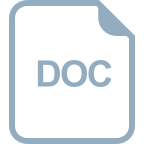
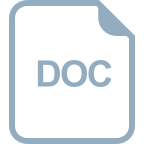
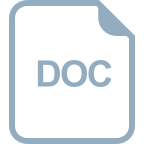
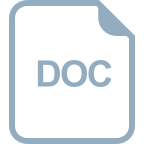





