linux开发进程间通信
时间: 2024-06-13 12:05:55 浏览: 109
Linux中有多种进程间通信的机制,包括管道、命名管道、信号量、消息队列、共享内存和套接字等。下面是其中几种机制的介绍和示例:
1. 管道(Pipe):管道是一种半双工的通信方式,数据只能单向流动,而且只能在具有亲缘关系的进程间使用。在Linux中,管道分为匿名管道和命名管道两种。其中,匿名管道只能在具有亲缘关系的进程间使用,而命名管道则可以在不具有亲缘关系的进程间使用。
示例代码:
```python
# 创建匿名管道
import os
r, w = os.pipe() # 返回读端和写端的文件描述符
# 在子进程中写入数据
pid = os.fork()
if pid == 0:
os.close(r)
w = os.fdopen(w, 'w')
w.write('Hello, parent process!')
w.close()
exit(0)
# 在父进程中读取数据
os.close(w)
r = os.fdopen(r)
print(r.read())
r.close()
```
2. 信号量(Semaphore):信号量是一种计数器,用于多进程之间的同步和互斥。在Linux中,信号量由一个非负整数和一个等待队列组成。
示例代码:
```python
import threading
# 创建信号量
semaphore = threading.Semaphore(0)
# 在子线程中释放信号量
def child_thread():
print('Child thread is running...')
semaphore.release()
# 在主线程中等待信号量
print('Main thread is waiting...')
semaphore.acquire()
print('Main thread got the semaphore!')
```
3. 共享内存(Shared Memory):共享内存是一种最快的进程间通信方式,它允许多个进程访问同一块内存区域。在Linux中,共享内存需要使用shmget、shmat和shmdt等系统调用。
示例代码:
```python
import os
import mmap
# 创建共享内存
fd = os.open('test.txt', os.O_RDWR | os.O_CREAT)
os.write(fd, b'Hello, world!')
size = os.path.getsize('test.txt')
mem = mmap.mmap(fd, size)
# 在子进程中修改共享内存
pid = os.fork()
if pid == 0:
mem[0:5] = b'Hi, '
mem.flush()
exit(0)
# 在父进程中读取共享内存
os.waitpid(pid, 0)
print(mem[:])
mem.close()
os.close(fd)
```
阅读全文
相关推荐
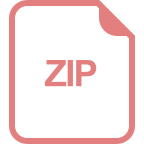
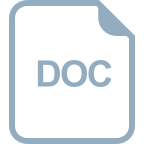
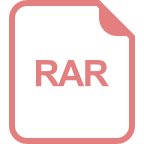
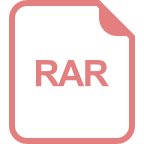


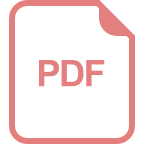


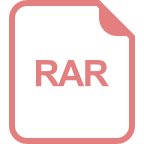
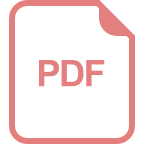
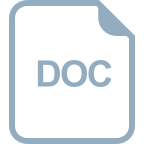
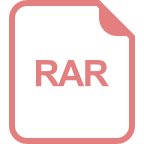

