gstreamer播放rtsp视频流 c++
时间: 2024-10-29 09:04:00 浏览: 68
GStreamer是一个强大的跨平台多媒体框架,用于处理音频、视频和数据流。在C++中利用GStreamer播放RTSP(Real-Time Streaming Protocol)视频流,你需要做以下几个步骤:
1. **安装Gstreamer库**:首先确保你在系统上已经安装了Gstreamer和其插件,包括支持RTSP的插件如gstreamer-plugins-good。
2. **构建Gstreamer pipeline**:Gstreamer的pipeline(管道)是一种描述媒体处理过程的方式。对于RTSP源,通常会包含`rtspsrc`元素作为输入,然后接上解码器、视频渲染等其他元素。例如:
```cpp
std::string pipeline = "rtspsrc location=<your_rtspsrc_url> ! decryptsrc ! rtph264depay ! h264parse ! decodebin ! videoconvert ! videorate ! ximagesink";
```
替换 `<your_rtspsrc_url>` 为实际的RTSP地址。
3. **创建Gstreamer上下文**:初始化一个Gstreamer上下文,并创建一个pipeline实例。
4. **设置回调函数**:Gstreamer通过信号槽机制处理事件,比如`new-sample`信号表示有新的视频帧到来,你可以在此处处理并显示视频帧。
5. **启动和停止 pipeline**:调用` gst_element_set_state(element, GST_STATE_PLAYING)`开始播放,`gst_element_stop(element)`停止播放。
6. **错误处理**:处理可能出现的Gstreamer错误,确保程序健壮。
示例代码片段(简化版):
```cpp
#include <gio/gio.h>
#include <gst/gst.h>
GstElement *pipeline;
// ...
void on_new_sample(GstElement *source, gpointer user_data) {
GstSample *sample = static_cast<GstSample*>(user_data);
// 处理新样本,例如获取并显示视频帧
}
int main() {
// 初始化Gstreamer上下文...
pipeline = gst_pipeline_new("playback");
// 添加pipeline元素到pipeline...
GstPadProbeReturn ret = gst_element_add_pad_probe(pipeline, ...,
(GstPadProbeCallback)on_new_sample, &sample, NULL);
if (ret != GST_PAD_PROBE_OK) {
// 错误处理...
}
// 设置状态...
// ...
// 等待用户交互结束...
// ...
// 停止并删除pipeline
gst_element_set_state(pipeline, GST_STATE_NULL);
gst_object_unref(pipeline);
return 0;
}
```
阅读全文
相关推荐
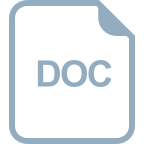
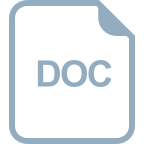
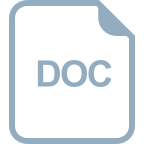
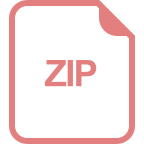

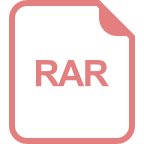
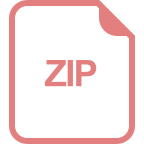

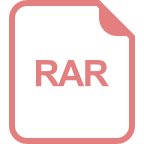
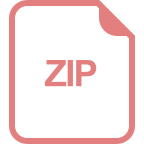
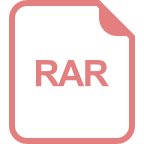
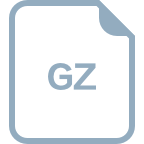
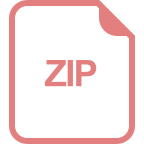
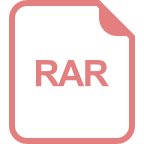
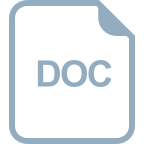
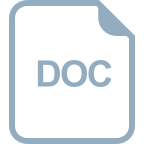

