C++ 编写一个多线程的gstreamer管道,分别用于rtsp推流、转发
时间: 2024-03-09 12:46:37 浏览: 26
好的,以下是一个简单的多线程 gstreamer 管道的示例,其中包括一个用于 RTSP 推流的管道和一个用于转发的管道。
```c++
#include <gst/gst.h>
#include <pthread.h>
GMainLoop *main_loop;
void *rtsp_pipeline(void *arg) {
GstElement *pipeline;
GstBus *bus;
GstMessage *msg;
/* 创建 GStreamer 管道 */
pipeline = gst_parse_launch("rtspsrc location=rtsp://your_rtsp_url ! rtph264depay ! h264parse ! video/x-h264,stream-format=avc ! rtph264pay name=pay0 pt=96 ! udpsink host=127.0.0.1 port=5000", NULL);
/* 启动管道 */
gst_element_set_state(pipeline, GST_STATE_PLAYING);
/* 监听管道消息 */
bus = gst_element_get_bus(pipeline);
msg = gst_bus_timed_pop_filtered(bus, GST_CLOCK_TIME_NONE, GST_MESSAGE_ERROR | GST_MESSAGE_EOS);
/* 处理管道消息 */
if (msg != NULL) {
gst_message_unref(msg);
}
gst_object_unref(bus);
gst_element_set_state(pipeline, GST_STATE_NULL);
gst_object_unref(pipeline);
return NULL;
}
void *forward_pipeline(void *arg) {
GstElement *pipeline;
GstBus *bus;
GstMessage *msg;
/* 创建 GStreamer 管道 */
pipeline = gst_parse_launch("udpsrc port=5000 ! application/x-rtp, payload=96 ! rtph264depay ! h264parse ! video/x-h264,stream-format=avc ! rtph264pay name=pay0 pt=96 ! udpsink host=127.0.0.1 port=5001", NULL);
/* 启动管道 */
gst_element_set_state(pipeline, GST_STATE_PLAYING);
/* 监听管道消息 */
bus = gst_element_get_bus(pipeline);
msg = gst_bus_timed_pop_filtered(bus, GST_CLOCK_TIME_NONE, GST_MESSAGE_ERROR | GST_MESSAGE_EOS);
/* 处理管道消息 */
if (msg != NULL) {
gst_message_unref(msg);
}
gst_object_unref(bus);
gst_element_set_state(pipeline, GST_STATE_NULL);
gst_object_unref(pipeline);
return NULL;
}
int main(int argc, char *argv[]) {
pthread_t rtsp_thread, forward_thread;
/* 初始化 GStreamer */
gst_init(&argc, &argv);
/* 创建主事件循环 */
main_loop = g_main_loop_new(NULL, FALSE);
/* 启动 RTSP 推流线程 */
pthread_create(&rtsp_thread, NULL, rtsp_pipeline, NULL);
/* 启动转发线程 */
pthread_create(&forward_thread, NULL, forward_pipeline, NULL);
/* 进入主事件循环 */
g_main_loop_run(main_loop);
/* 等待线程结束 */
pthread_join(rtsp_thread, NULL);
pthread_join(forward_thread, NULL);
/* 清理资源 */
g_main_loop_unref(main_loop);
gst_deinit();
return 0;
}
```
这个示例创建了两个线程,分别用于启动 RTSP 推流管道和转发管道。在管道中,我们使用 GStreamer 的元素来处理视频流,例如 `rtspsrc` 用于从 RTSP URL 获取视频流,`udpsink` 用于将视频流发送到指定的 IP 地址和端口号。在管道启动后,我们使用 GStreamer 的总线机制来监听管道消息,以便在错误或者结束时进行处理。
需要注意的是,这个示例是一个简单的多线程 gstreamer 管道,实际使用中可能需要更加细致的调整和优化。同时,还需要注意线程间的同步和资源管理,以避免出现竞态条件等问题。
相关推荐
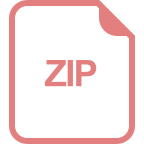
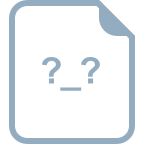














