迷宫寻宝ai竞技赛必胜代码
时间: 2024-08-15 16:03:45 浏览: 86
迷宫寻宝AI竞技赛通常涉及到路径搜索算法,如A*算法、Dijkstra算法或深度优先搜索(DFS)等。为了编写一种可能的“必胜”代码,假设我们使用A*算法,因为它的效率较高,而且能够找到从起点到终点的最短路径。
```python
import heapq
# 定义网格地图数据结构
class Grid:
def __init__(self, width, height, start, goal):
self.width = width
self.height = height
self.start = start
self.goal = goal
self.grid = [ * width for _ in range(height)]
# ...
def a_star_search(grid, heuristic_func):
open_set = [(grid[start][start], (start, start), None)]
came_from = {}
g_score = {start: 0}
f_score = {start: heuristic_func(start)}
while open_set:
current_distance, current_pos, parent_pos = heapq.heappop(open_set)
if current_pos == grid.goal:
path = [current_pos]
while parent_pos is not None:
path.append(parent_pos)
parent_pos = came_from[parent_pos]
return path[::-1]
for dx, dy in [(0, 1), (0, -1), (1, 0), (-1, 0)]:
next_x, next_y = current_pos + dx, current_pos + dy
# 检查边界和墙壁
if (
0 <= next_x < grid.width
and 0 <= next_y < grid.height
and grid.grid[next_y][next_x] == 0
):
tentative_g_score = g_score[current_pos] + 1
if next_pos not in g_score or tentative_g_score < g_score[next_pos]:
came_from[next_pos] = current_pos
g_score[next_pos] = tentative_g_score
f_score[next_pos] = g_score[next_pos] + heuristic_func(next_pos)
heapq.heappush(open_set, (f_score[next_pos], next_pos, current_pos))
# 如果没有找到路径,则返回None
return None
# 使用曼哈顿距离作为启发式函数
heuristic_func = lambda pos: abs(pos - grid.goal) + abs(pos - grid.goal)
# 初始化并运行A*搜索
path = a_star_search(your_grid_instance, heuristic_func)
if path:
print("必胜代码找到了一条路径:", path)
else:
print("无法保证必胜,可能存在无解或路径复杂的情况。")
```
阅读全文
相关推荐
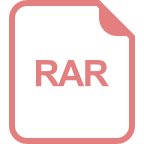
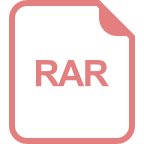

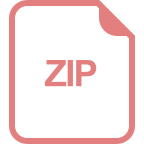
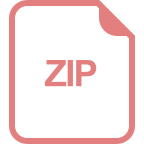
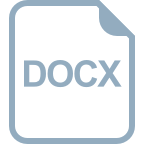
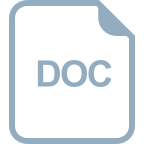
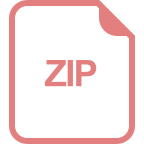
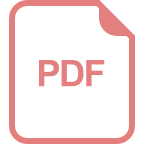
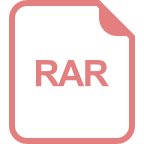
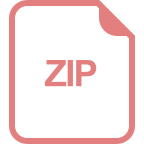
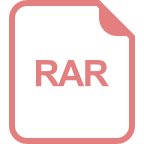
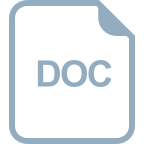