ai创想家迷宫寻宝的必胜代码
时间: 2024-10-25 07:14:10 浏览: 141
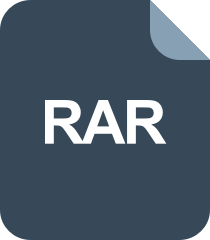
迷宫寻宝源码

"AI创想家迷宫寻宝"通常是指一种基于人工智能算法的游戏,玩家需要通过编程让角色智能地寻找宝藏。这里并没有特定的"必胜代码",因为游戏策略依赖于对迷宫结构的理解、路径搜索算法(如深度优先搜索、广度优先搜索或A*算法)、以及决策制定等。
一个简单的Python示例,使用递归的深度优先搜索(DFS)可能会这样设计:
```python
def find_path(maze, start, end):
def dfs(current):
if current == end:
return True
for next_step in maze[current]:
if dfs(next_step):
return True
return False
return dfs(start)
# 假设maze是一个二维列表,每个元素表示一个位置,0表示墙,非0表示可以走的步数
maze = [[1, 1, 0], [1, 0, 1], [0, 1, 1]]
start = (0, 0)
end = (len(maze) - 1, len(maze[0]) - 1)
if find_path(maze, start, end):
print("找到了胜利之路!")
else:
print("无法找到通往终点的路。")
```
阅读全文
相关推荐
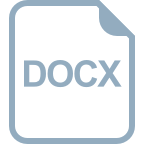
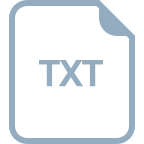


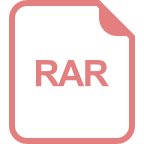
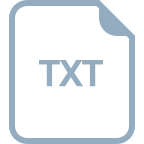
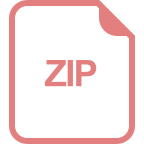
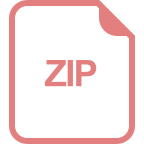
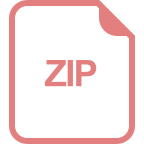
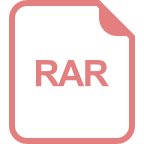
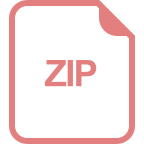
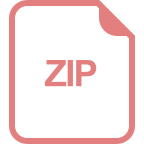
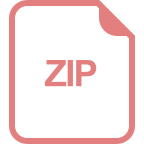
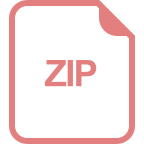
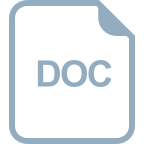