1、定义函数,判断一个字符串中是否既有数字又有字母 2、定义函数,统计一个列表中各个字符出现的次数 3、定义函数,对整数列表数据按奇偶排序,偶数在前,奇数在后
时间: 2024-12-23 10:17:38 浏览: 14
1. 在Python中,你可以创建两个函数来分别实现这两个功能:
**检查字符串:**
```python
def has_numbers_and_letters(s):
has_num = any(char.isdigit() for char in s)
has_alpha = any(char.isalpha() for char in s)
return has_num and has_alpha
# 测试函数
print(has_numbers_and_letters("abc123")) # 输出:True
print(has_numbers_and_letters("12345")) # 输出:False
```
**统计字符出现次数:**
```python
def count_chars(lst):
char_count = {}
for item in lst:
for char in item:
if char in char_count:
char_count[char] += 1
else:
char_count[char] = 1
return char_count
# 测试函数
test_list = ['apple', 'banana']
print(count_chars(test_list)) # 输出:{'a': 6, 'p': 4, 'l': 2, 'e': 2, 'b': 1, 'n': 3}
```
**按奇偶排序整数列表:**
```python
def sort_even_then_odd(numbers):
even_nums = [num for num in numbers if num % 2 == 0]
odd_nums = [num for num in numbers if num % 2 != 0]
return sorted(even_nums) + sorted(odd_nums)
# 测试函数
nums = [1, 3, 2, 5, 4]
print(sort_even_then_odd(nums)) # 输出:[2, 4, 1, 3, 5]
```
阅读全文
相关推荐
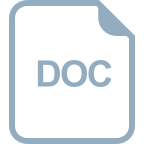
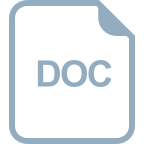
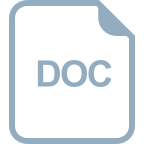
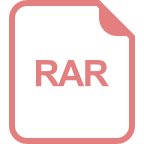
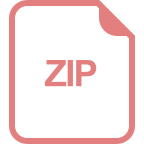
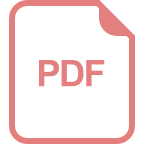
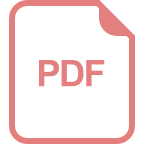
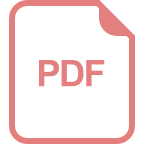
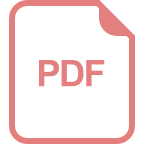
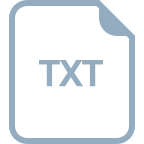
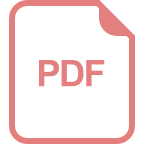
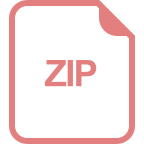
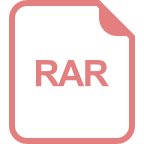
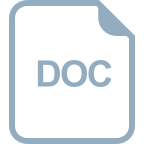
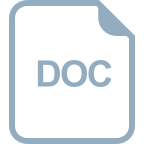