C#窗体程序,实现图片的拖拽,放大,缩小,旋转的完整代码
时间: 2024-10-20 09:08:55 浏览: 27
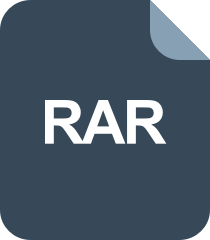
C#图片放大,缩小,移动

在C#中创建一个Windows Form应用程序,实现图片的拖放、缩放、放大和旋转功能需要一些步骤,这里我会给出一个简化的示例代码框架。首先,你需要添加`System.Drawing.dll`库,然后按照以下代码结构编写:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
using System.Runtime.InteropServices;
public partial class MainForm : Form
{
private PictureBox pictureBox1; // 图片框控件
private bool isDragging = false; // 是否正在拖动
private Point dragStartPoint; // 拖动开始点
[DllImport("gdi32.dll")]
private static extern IntPtr GetDC(IntPtr hWnd);
[DllImport("gdi32.dll")]
private static extern bool ReleaseDC(IntPtr hWnd, IntPtr hdc);
//...其他Form的构造函数和加载事件...
protected override void OnLoad(EventArgs e)
{
InitializeComponent();
pictureBox1 = new PictureBox();
pictureBox1.Dock = DockStyle.Fill;
pictureBox1.SizeMode = PictureBoxSizeMode.Zoom;
pictureBox1.ImageLocation = "your_image_path.jpg"; // 替换为你的图片路径
Controls.Add(pictureBox1);
}
protected override void OnMouseDown(MouseEventArgs e)
{
if (pictureBox1.Contains(e.Location))
isDragging = true;
dragStartPoint = e.Location;
}
protected override void OnMouseMove(MouseEventArgs e)
{
if (isDragging)
{
// 缩放和移动图片
var delta = e.Location - dragStartPoint;
var newWidth = pictureBox1.Width + delta.X;
var newHeight = pictureBox1.Height + delta.Y;
pictureBox1.Size = new Size(Math.Max(pictureBox1.MinimumSize.Width, newWidth), Math.Max(pictureBox1.MinimumSize.Height, newHeight));
// 旋转图片需要更复杂的计算,这里仅提供基础示例
var rotationAngle = CalculateRotationAngle(delta);
RotateImage(rotationAngle); // 自定义方法来旋转图片
dragStartPoint = e.Location;
}
}
protected override void OnMouseUp(MouseEventArgs e)
{
isDragging = false;
}
private float CalculateRotationAngle(Point delta)
{
// 这里只是一个简单的假设,实际的旋转角度计算可能会更复杂
return delta.X > delta.Y ? Math.PI / 4 * 3 : Math.PI / 4;
}
private void RotateImage(float angle)
{
using (var graphics = Graphics.FromImage(pictureBox1.Image))
{
graphics.TranslateTransform(pictureBox1.ClientRectangle.Left, pictureBox1.ClientRectangle.Top);
graphics.RotateTransform(angle);
graphics.TranslateTransform(-pictureBox1.ClientRectangle.Left, -pictureBox1.ClientRectangle.Top);
pictureBox1.Invalidate();
}
}
// ...其他必要的事件处理和其他业务逻辑...
}
//
阅读全文
相关推荐
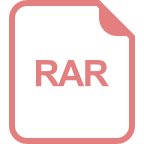
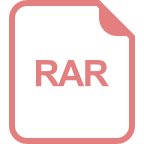


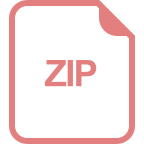
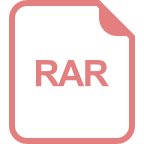
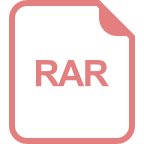
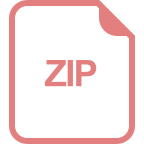
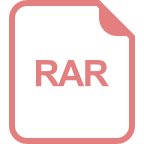
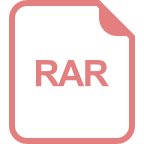
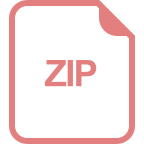
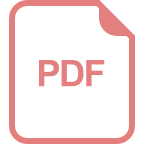
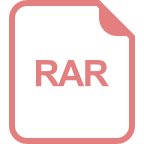
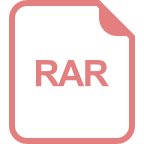
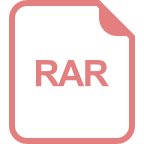
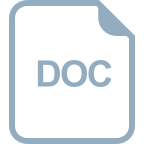
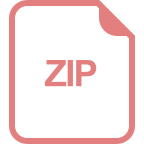