def mymodel(): inputs = keras.Input(shape=(x_train.shape[1], x_train.shape[2])) h1 = layers.Conv1D(filters=8, kernel_size=3, strides=1, padding='same', activation='relu')(inputs) h1 = layers.MaxPool1D(pool_size=2, strides=2, padding='same')(h1) h1 = layers.Conv1D(filters=16, kernel_size=3, strides=1, padding='same', activation='relu')(h1) h1 = layers.MaxPool1D(pool_size=2, strides=2, padding='same')(h1) h1 = layers.Flatten()(h1) h1 = layers.Dropout(0.6)(h1) h1 = layers.Dense(32, activation='relu')(h1) h1 = layers.Dense(10, activation='softmax')(h1) deep_model = keras.Model(inputs, h1, name="cnn") return deep_model model = mymodel() model.summary()#把整个网络打印出来 startdate = datetime.utcnow() # 获取当前时间 # 编译模型 model.compile( optimizer=keras.optimizers.Adam(), loss='sparse_categorical_crossentropy', metrics=['accuracy']) history = model.fit(x_train, y_train, batch_size=512, epochs=100, verbose=1, validation_data=(x_valid, y_valid), callbacks=[CustomModelCheckpoint( model, r'best_fft_1dcnn_512.h5')])定义的模型训练好后怎么加载使用
时间: 2024-04-10 21:33:26 浏览: 176
训练好的模型可以通过`load_model()`函数加载并使用。你可以按照以下步骤加载和使用模型:
1. 首先,导入`keras.models`模块:
```python
from keras.models import load_model
```
2. 使用`load_model()`函数加载模型,并将其保存在变量中:
```python
loaded_model = load_model('best_fft_1dcnn_512.h5')
```
3. 现在,你可以使用`loaded_model`变量来对新数据进行预测或评估:
```python
predictions = loaded_model.predict(new_data)
```
请注意,加载的模型将包含之前训练时保存的权重和模型架构。确保在加载模型时,模型架构与之前训练时的架构完全一致。
希望这个回答对你有帮助!如果你有任何其他问题,请随时提问。
相关问题
# 设置输入层节点数、隐层节点数 in_nodes=784 h1_nodes=100 h2_nodes=100 h3_nodes=50 # 定义输入、输出、prob的placeholder x=tf.placeholder(tf.float32,[None,in_nodes]) y_=tf.placeholder(tf.float32,[None,10]) prob=tf.placeholder(tf.float32) # 设置第一隐层 w1=weight(in_nodes, h1_nodes, 0.1, 0.005) b1=tf.Variable(tf.zeros([h1_nodes])) h1=tf.nn.relu(tf.matmul(x,w1)+b1) # 设置第二隐层 w2=weight(h1_nodes, h2_nodes, 0.1, 0.0) b2=tf.Variable(tf.zeros([h2_nodes])) h2=tf.nn.relu(tf.matmul(h1,w2)+b2) h2_drop=tf.nn.dropout(h2, prob) # 设置第三隐层 w3=weight(h2_nodes, h3_nodes, 0.1, 0.0) b3=tf.Variable(tf.zeros([h3_nodes])) h3=tf.nn.relu(tf.matmul(h2_drop,w3)+b3) h3_drop=tf.nn.dropout(h3, prob) # 设置softmax输出层 w4=weight(h3_nodes, 10, 0.1, 0.0) b4=tf.Variable(tf.zeros([10])) y=tf.nn.softmax(tf.matmul(h3_drop,w4)+b4)
将上述代码改写成 TensorFlow 2.x 版本的代码如下:
```python
import tensorflow as tf
def weight(shape, stddev, wd):
initial = tf.random.truncated_normal(shape, stddev=stddev)
var = tf.Variable(initial)
if wd is not None:
weight_decay = tf.multiply(tf.nn.l2_loss(var), wd, name='weight_loss')
tf.add_to_collection('losses', weight_decay)
return var
class MyModel(tf.keras.Model):
def __init__(self, in_nodes=784, h1_nodes=100, h2_nodes=100, h3_nodes=50):
super(MyModel, self).__init__()
self.w1 = weight([in_nodes, h1_nodes], 0.1, 0.005)
self.b1 = tf.Variable(tf.zeros([h1_nodes]))
self.w2 = weight([h1_nodes, h2_nodes], 0.1, 0.0)
self.b2 = tf.Variable(tf.zeros([h2_nodes]))
self.w3 = weight([h2_nodes, h3_nodes], 0.1, 0.0)
self.b3 = tf.Variable(tf.zeros([h3_nodes]))
self.w4 = weight([h3_nodes, 10], 0.1, 0.0)
self.b4 = tf.Variable(tf.zeros([10]))
def call(self, inputs, prob):
x = inputs
y_ = tf.cast(inputs, tf.float32)
h1 = tf.nn.relu(tf.matmul(x, self.w1) + self.b1)
h2 = tf.nn.relu(tf.matmul(h1, self.w2) + self.b2)
h2_drop = tf.nn.dropout(h2, rate=prob)
h3 = tf.nn.relu(tf.matmul(h2_drop, self.w3) + self.b3)
h3_drop = tf.nn.dropout(h3, rate=prob)
y = tf.nn.softmax(tf.matmul(h3_drop, self.w4) + self.b4)
return y
model = MyModel()
x = tf.keras.Input(shape=(None, 784))
prob = tf.keras.Input(shape=())
y = model(x, prob)
y_ = tf.keras.Input(shape=(None, 10))
# 定义损失函数
cross_entropy = tf.reduce_mean(-tf.reduce_sum(y_ * tf.math.log(y), reduction_indices=[1]))
tf.add_to_collection('losses', cross_entropy)
loss = tf.add_n(tf.get_collection('losses'))
# 定义优化器
train_step = tf.train.AdamOptimizer(1e-4).minimize(loss)
# 训练模型
with tf.Session() as sess:
sess.run(tf.global_variables_initializer())
for i in range(1000):
batch_xs, batch_ys = mnist.train.next_batch(100)
sess.run(train_step, feed_dict={x: batch_xs, y_: batch_ys, prob: 0.5})
```
在 TensorFlow 2.x 中,可以使用 `tf.reduce_mean` 和 `tf.reduce_sum` 函数来计算张量的平均值和总和;使用 `tf.math.log` 函数来计算张量的自然对数。此外,可以使用 `tf.train.AdamOptimizer` 来定义优化器,使用 `model.trainable_variables` 来获取所有可训练的变量。
keras搭建神经网络
Keras是一个基于Python的深度学习库,提供了许多便捷的API用于神经网络的搭建。Keras框架的特点是高度模块化、易于扩展、支持GPU和CPU的混合计算、用户友好,可以方便的构建各种神经网络模型并进行训练和预测。
在Keras中搭建神经网络,首先需要确定神经网络的模型。Keras支持多种模型构建方法,包括序列模型、函数式模型和子类化API等。
序列模型是最简单的一种,它是一个线性的神经网络模型,是多个网络层的线性堆叠,其中的每一层都是前一层的输出作为下一层的输入。可以用以下方式构建一个序列模型:
```
from keras.models import Sequential
from keras.layers import Dense
model = Sequential()
model.add(Dense(units=32, activation='relu', input_dim=100))
model.add(Dense(units=10, activation='softmax'))
```
函数式模型可以用于构建更复杂的模型,如多输入和多输出的神经网络。可以用以下方式构建一个函数式模型:
```
from keras.layers import Input, Dense
from keras.models import Model
# This returns a tensor
inputs = Input(shape=(784,))
# a layer instance is callable on a tensor, and returns a tensor
x = Dense(64, activation='relu')(inputs)
x = Dense(64, activation='relu')(x)
predictions = Dense(10, activation='softmax')(x)
# This creates a model that includes
# the Input layer and three Dense layers
model = Model(inputs=inputs, outputs=predictions)
model.compile(optimizer='rmsprop',
loss='categorical_crossentropy',
metrics=['accuracy'])
```
子类化API提供了更加灵活的构建方式,可以通过自定义网络层和模型的方式实现复杂的神经网络。可以用以下方式构建一个子类化API模型:
```
import tensorflow as tf
from tensorflow import keras
from tensorflow.keras import layers
class MyModel(keras.Model):
def __init__(self):
super(MyModel, self).__init__()
self.dense1 = layers.Dense(64, activation='relu')
self.dense2 = layers.Dense(64, activation='relu')
self.dense3 = layers.Dense(10, activation='softmax')
def call(self, inputs):
x = self.dense1(inputs)
x = self.dense2(x)
x = self.dense3(x)
return x
model = MyModel()
model.compile(optimizer=keras.optimizers.Adam(learning_rate=1e-3),
loss=keras.losses.SparseCategoricalCrossentropy(),
metrics=[keras.metrics.SparseCategoricalAccuracy()])
```
无论采用何种方式搭建神经网络,都需要进行模型的编译和训练。模型的编译需要指定优化器、损失函数和评估指标。模型的训练则需要指定训练集、验证集、批处理大小和训练轮数等参数。可以用以下方式训练和评估模型:
```
history = model.fit(x_train, y_train,
batch_size=64,
epochs=5,
validation_data=(x_val, y_val))
test_scores = model.evaluate(x_test, y_test, verbose=2)
print('Test loss:', test_scores[0])
print('Test accuracy:', test_scores[1])
```
以上是Keras搭建神经网络的基本流程,需要根据具体问题和数据集的不同进行调整和优化。
阅读全文
相关推荐
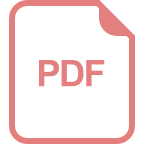
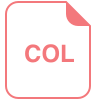
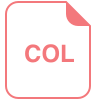
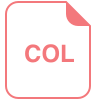
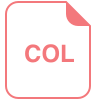
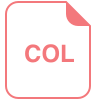
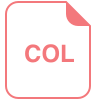
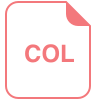
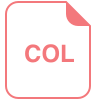
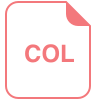
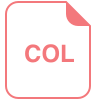
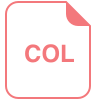
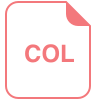
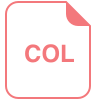
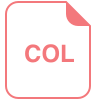
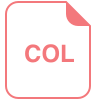