linux c 串口 demo
时间: 2023-12-15 15:02:40 浏览: 35
Linux C 串口 demo 可以用来演示如何在 Linux 系统中使用 C 语言编写串口通信的程序。以下是一个简单的例子:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#include <termios.h>
int main()
{
int fd;
struct termios options;
// 打开串口设备
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY);
if (fd == -1) {
perror("无法打开串口设备");
exit(EXIT_FAILURE);
}
// 配置串口参数
tcgetattr(fd, &options);
cfsetispeed(&options, B4800);
cfsetospeed(&options, B4800);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
tcsetattr(fd, TCSANOW, &options);
// 发送数据
char buffer[] = "Hello, serial port!";
write(fd, buffer, sizeof(buffer));
// 接收数据
char read_buffer[255];
ssize_t num_read = read(fd, &read_buffer, sizeof(read_buffer));
if (num_read > 0) {
printf("接收到的数据:%s\n", read_buffer);
}
// 关闭串口设备
close(fd);
return 0;
}
```
上述代码首先打开串口设备 `/dev/ttyS0`,如果打开失败会输出错误信息并退出程序。然后,通过调用 `tcgetattr()` 和 `tcsetattr()` 函数来配置串口参数,其中包括波特率、数据位、奇偶校验、停止位等。接着,使用 `write()` 函数向串口发送数据,本例中发送的数据为 "Hello, serial port!"。最后,使用 `read()` 函数从串口接收数据,并打印出接收到的数据。
这个简单的串口 demo 可以作为参考,帮助你进一步了解 Linux C 串口编程的基本知识和使用方法。实际应用中,你可能还需要处理串口的异常情况、使用缓冲区来读写数据等更多的功能。
相关推荐
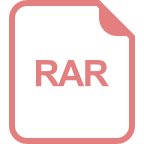














