用java复制文件使用字节流将a.txt中的内容复制到b.txt。要求:a.txt请自行创建,复制过程中,包含必要的错误检查,如检查源文件是否存在。
时间: 2024-10-19 08:04:04 浏览: 27
在Java中,我们可以使用`Files.copy()`方法结合`ByteChannel`来复制文件。首先,我们需要检查源文件(a.txt)是否存在,然后打开源文件和目标文件的通道,并进行实际的数据复制。以下是一个完整的示例:
```java
import java.io.*;
import java.nio.channels.FileChannel;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class FileCopy {
public static void main(String[] args) {
Path sourceFilePath = Paths.get("a.txt"); // 指定源文件路径
Path targetFilePath = Paths.get("b.txt"); // 指定目标文件路径
try {
// 检查源文件是否存在
if (!Files.exists(sourceFilePath)) {
throw new FileNotFoundException("Source file does not exist: " + sourceFilePath);
}
// 打开源文件和目标文件通道
FileInputStream fis = new FileInputStream(sourceFilePath.toFile());
FileOutputStream fos = new FileOutputStream(targetFilePath.toFile());
// 获取通道
FileChannel inChannel = fis.getChannel();
FileChannel outChannel = fos.getChannel();
// 尝试复制文件
long copiedBytes = Files.copy(inChannel, outChannel);
// 关闭通道和文件流
inChannel.close();
outChannel.close();
fis.close();
fos.close();
System.out.printf("%d bytes have been copied from %s to %s.%n", copiedBytes, sourceFilePath, targetFilePath);
} catch (IOException e) {
System.err.println("Error copying file: " + e.getMessage());
}
}
}
```
在这个例子中,我们首先创建了`FileInputStream`和`FileOutputStream`来获取源文件和目标文件的通道。然后使用`Files.copy()`方法进行数据复制,并打印出复制的字节数。如果有任何I/O错误,我们将抛出自定义的异常。
请注意,你需要先创建好`a.txt`文件才能运行此程序。
阅读全文
相关推荐
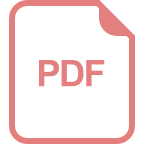
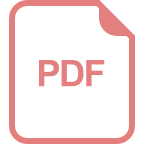
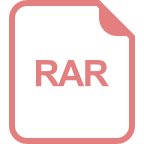
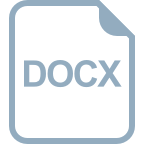
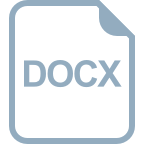
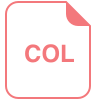
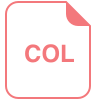
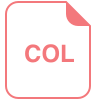
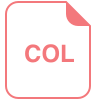
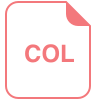
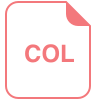
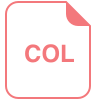
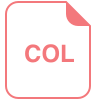
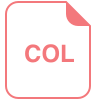
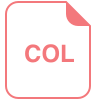
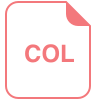
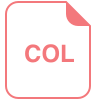
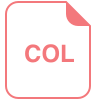