yolov8 flask部署
时间: 2023-12-27 17:25:39 浏览: 257
以下是使用Flask框架进行YOLOv8模型部署的示例代码:
```python
from flask import Flask, request, jsonify
import cv2
import numpy as np
app = Flask(__name__)
# 加载YOLOv8模型
net = cv2.dnn.readNetFromDarknet('yolov8.cfg', 'yolov8.weights')
layer_names = net.getLayerNames()
output_layers = [layer_names[i[0] - 1] for i in net.getUnconnectedOutLayers()]
# 定义目标类别
classes = ['class1', 'class2', 'class3']
@app.route('/predict', methods=['POST'])
def predict():
# 获取上传的图片
image = request.files['image']
image.save('input.jpg')
# 进行目标检测
img = cv2.imread('input.jpg')
height, width, channels = img.shape
blob = cv2.dnn.blobFromImage(img, 0.00392, (416, 416), (0, 0, 0), True, crop=False)
net.setInput(blob)
outs = net.forward(output_layers)
class_ids = []
confidences = []
boxes = []
for out in outs:
for detection in out:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5:
center_x = int(detection[0] * width)
center_y = int(detection[1] * height)
w = int(detection[2] * width)
h = int(detection[3] * height)
x = int(center_x - w / 2)
y = int(center_y - h / 2)
boxes.append([x, y, w, h])
confidences.append(float(confidence))
class_ids.append(class_id)
# 绘制边界框和标签
indexes = cv2.dnn.NMSBoxes(boxes, confidences, 0.5, 0.4)
font = cv2.FONT_HERSHEY_PLAIN
colors = np.random.uniform(0, 255, size=(len(classes), 3))
for i in range(len(boxes)):
if i in indexes:
x, y, w, h = boxes[i]
label = str(classes[class_ids[i]])
color = colors[class_ids[i]]
cv2.rectangle(img, (x, y), (x + w, y + h), color, 2)
cv2.putText(img, label, (x, y + 30), font, 3, color, 3)
cv2.imwrite('output.jpg', img)
# 返回结果
result = {
'image': 'output.jpg',
'detections': len(boxes)
}
return jsonify(result)
if __name__ == '__main__':
app.run()
```
请确保已经安装了Flask和OpenCV库,并将YOLOv8的配置文件(yolov8.cfg)和权重文件(yolov8.weights)放在同一目录下。运行上述代码后,可以通过发送POST请求到`http://localhost:5000/predict`来进行目标检测。请求中需要包含一个名为`image`的文件字段,值为待检测的图片文件。
阅读全文
相关推荐
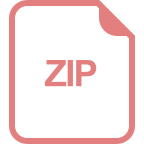
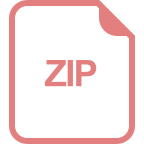
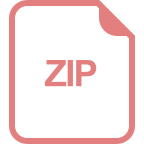

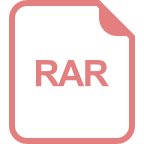

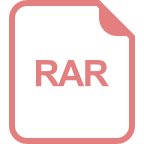
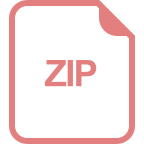
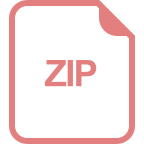
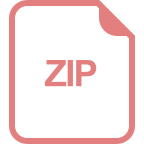
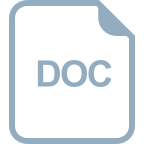
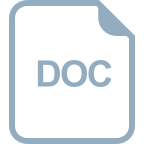
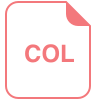



