c++遍历删除unordered_map
时间: 2023-12-19 14:31:51 浏览: 44
以下是C++遍历删除unordered_map的例子:
1. 使用迭代器遍历删除unordered_map
```cpp
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<int, int> myMap = {{1, 10}, {2, 20}, {3, 30}, {4, 40}, {5, 50}};
int target = 3;
for (auto it = myMap.begin(); it != myMap.end();) {
if (it->first == target) {
it = myMap.erase(it);
} else {
++it;
}
}
for (const auto& pair : myMap) {
std::cout << pair.first << " : " << pair.second << std::endl;
}
return 0;
}
```
2. 使用C++11的范围for循环遍历删除unordered_map
```cpp
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<int, int> myMap = {{1, 10}, {2, 20}, {3, 30}, {4, 40}, {5, 50}};
int target = 3;
for (auto it = myMap.begin(); it != myMap.end();) {
if (it->first == target) {
it = myMap.erase(it);
} else {
++it;
}
}
for (const auto& pair : myMap) {
std::cout << pair.first << " : " << pair.second << std::endl;
}
return 0;
}
```
相关推荐
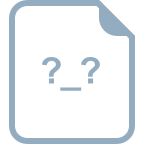
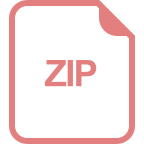














