凯撒python解密算法
时间: 2023-09-29 16:09:16 浏览: 76
凯撒解密算法可以通过对密文中的每个字符进行向前推几个字母的操作,得到原始的明文。代码如下:
```python
def jiema(m):
for i in m:
if i == ' ':
return " "
elif 97 <= ord(i) < 123 - key or 65 <= ord(i) < 91 - key:
m = chr(ord(i) - key)
return m
elif 97 < ord(i) < 97 + key or 65 <= ord(i) < 65 + key:
m = chr(ord(i) - key + 26)
return m
m = input('请输入密文:')
out = ''
for key in range(1, 27):
for n in list(map(jiema, m)):
out = out + str(n)
print('第%d个可能的结果是%s' % (key, out))
out = ''
```
以上代码通过遍历所有可能的位移值,并对每个位移值进行解密操作,最终输出所有可能的解密结果。
相关问题
python凯撒密码加密算法
凯撒密码是一种古老的加密算法,它通过将明文中的每个字母按照一定的规则替换成密文中的字母来实现加密。下面是使用Python实现凯撒密码加密算法的代码:
```python
def caesar_encrypt(plaintext, key):
ciphertext = ""
for char in plaintext:
if char.isalpha():
if char.isupper():
ciphertext += chr((ord(char) + key - 65) % 26 + 65)
else:
ciphertext += chr((ord(char) + key - 97) % 26 + 97)
else:
ciphertext += char
return ciphertext
```
其中,`plaintext`是要加密的明文,`key`是密钥,表示每个字母向前移动的位数。这个函数会返回加密后的密文。
下面是一个例子,演示如何使用这个函数加密一个字符串:
```python
plaintext = "Hello, World!"
key = 3
ciphertext = caesar_encrypt(plaintext, key)
print(ciphertext) # 输出:Khoor, Zruog!
```
这个例子中,我们将明文字符串`"Hello, World!"`加密成了密文字符串`"Khoor, Zruog!"`,密钥为3。
凯撒密码加密算法python
以下是Python实现的凯撒密码加密算法:
```python
def caesar_cipher(text, shift):
"""
凯撒密码加密算法
:param text: 明文字符串
:param shift: 移位数,可以是正数或负数
:return: 密文字符串
"""
cipher_text = ""
for char in text:
# 如果是大写字母
if char.isupper():
cipher_text += chr((ord(char) + shift - 65) % 26 + 65)
# 如果是小写字母
elif char.islower():
cipher_text += chr((ord(char) + shift - 97) % 26 + 97)
else:
# 非字母字符不加密
cipher_text += char
return cipher_text
```
使用示例:
```python
text = "Hello, World!"
shift = 3
cipher_text = caesar_cipher(text, shift)
print(cipher_text) # 输出:Khoor, Zruog!
# 解密
plain_text = caesar_cipher(cipher_text, -shift)
print(plain_text) # 输出:Hello, World!
```
注意:凯撒密码是一种非常简单的加密算法,容易被破解,不适用于安全性要求较高的场合。
阅读全文
相关推荐
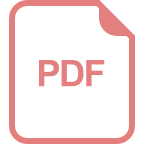
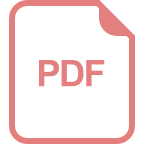
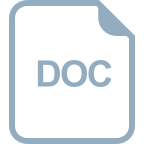







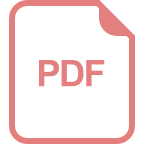
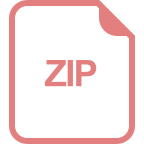



