cur 是一个链表 cur.val 什么意思
时间: 2024-10-08 15:26:17 浏览: 39
`cur` 一般在描述链表(Linked List)的上下文中代表当前节点(current node),而 `cur.val` 则是指该节点的值(value)。在链表中,每个节点通常包含两个部分:一个是存储数据的域(value),另一个是链接到下一个节点的指针(next pointer)。
例如,假设有一个单向链表,`cur` 指向链表中的某一个位置,那么 `cur.val` 就是该位置节点存储的数据。这个表达式用于访问并读取链表当前节点的实际内容。
举个例子:
```python
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
# 创建一个链表
head = ListNode(1)
head.next = ListNode(2)
head.next.next = ListNode(3)
# 遍历链表,打印每个节点的值
current_node = head
while current_node:
print(current_node.val) # 输出:1, 2, 3
current_node = current_node.next
```
在这个例子中,`current_node` 是指针,每次循环它都会向前移动一次,`current_node.val` 就会指向并打印出下一个节点的值。
相关问题
cur=cur.next if cur.val==val2: tmp=ListNode(val1) tmp.next=cur.next cur.next=tmp
哦,您想问的是关于链表的操作吧?我可以理解为您是想把链表中值为val2的节点替换为值为val1的节点,并保持节点的相对位置不变。对吧?
如果是这样的话,您可以使用如下的代码实现:
```
while cur:
if cur.val == val2:
tmp = ListNode(val1)
tmp.next = cur.next
cur.next = tmp
break
cur = cur.next
```
其中,cur代表当前节点,val1代表要插入的新值,val2代表需要替换的旧值。代码中,我们遍历链表,找到值为val2的节点后,创建一个新节点tmp,并将cur.next赋值给它的next属性,将tmp赋值给cur.next属性,从而在链表中将值为val2的节点替换为了值为val1的节点。
希望我的回答能够帮助到您!
对下面代码每一步含义进行注释 def convert_to_doubly_linked_list(self): if not self.root: return None def convert(root): if not root.left and not root.right: return ListNode(root.val) if not root.left: right_head = convert(root.right) right_tail = right_head while right_tail.next: right_tail = right_tail.next cur_node = ListNode(root.val, None, right_head) right_head.prev = cur_node return cur_node if not root.right: left_tail = convert(root.left) left_head = left_tail while left_head.prev: left_head = left_head.prev cur_node = ListNode(root.val, left_tail, None) left_tail.next = cur_node return cur_node left_tail = convert(root.left) right_head = convert(root.right) left_head = left_tail while left_head.prev: left_head = left_head.prev right_tail = right_head while right_tail.next: right_tail = right_tail.next cur_node = ListNode(root.val, left_tail, right_head) left_tail.next = cur_node right_head.prev = cur_node return left_head return convert(self.root) def inorder_traversal(self, root): if not root: return self.inorder_traversal(root.left) print(root.val, end=' ') self.inorder_traversal(root.right) def print_bst(self): self.inorder_traversal(self.root) print() def traverse_doubly_linked_list(self, head): cur_node = head while cur_node: print(cur_node.val, end=' ') cur_node = cur_node.next print() def reverse_traverse_doubly_linked_list(self, head): cur_node = head while cur_node.next: cur_node = cur_node.next while cur_node: print(cur_node.val, end=' ') cur_node = cur_node.prev print()
这段代码是一个二叉搜索树(BST)转化为双向链表的函数,同时提供了打印BST和遍历双向链表的函数。
- `def convert_to_doubly_linked_list(self):`:定义一个将BST转化为双向链表的函数。
- `if not self.root:`:如果BST为空,则返回None。
- `def convert(root):`:定义一个递归函数,将BST转化为双向链表。
- `if not root.left and not root.right:`:如果该节点没有左右子树,返回一个只包含该节点值的ListNode。
- `if not root.left:`:如果该节点没有左子树,将右子树转化为双向链表,并将节点值作为新的头结点,返回该节点。
- `if not root.right:`:如果该节点没有右子树,将左子树转化为双向链表,并将节点值作为新的尾结点,返回该节点。
- `left_tail = convert(root.left)`:将左子树转化为双向链表,并返回左子树的尾结点。
- `right_head = convert(root.right)`:将右子树转化为双向链表,并返回右子树的头结点。
- `left_head = left_tail`:将左子树的头结点设置为左子树的尾结点。
- `while left_head.prev:`:找到左子树双向链表的头结点。
- `right_tail = right_head`:将右子树的尾结点设置为右子树的头结点。
- `while right_tail.next:`:找到右子树双向链表的尾结点。
- `cur_node = ListNode(root.val, left_tail, right_head)`:创建一个新的节点,值为当前节点值,左指针指向左子树双向链表的尾结点,右指针指向右子树双向链表的头结点。
- `left_tail.next = cur_node`:将左子树双向链表的尾结点的右指针指向新节点。
- `right_head.prev = cur_node`:将右子树双向链表的头结点的左指针指向新节点。
- `return left_head`:返回双向链表的头结点。
- `return convert(self.root)`:调用递归函数convert并返回结果。
- `def inorder_traversal(self, root):`:定义一个中序遍历BST的函数。
- `if not root:`:如果该节点为空,则返回。
- `self.inorder_traversal(root.left)`:递归遍历左子树。
- `print(root.val, end=' ')`:输出当前节点的值。
- `self.inorder_traversal(root.right)`:递归遍历右子树。
- `def print_bst(self):`:定义一个打印BST的函数。
- `self.inorder_traversal(self.root)`:调用中序遍历函数遍历BST。
- `print()`:输出一个空行。
- `def traverse_doubly_linked_list(self, head):`:定义一个遍历双向链表的函数。
- `cur_node = head`:将当前节点指向链表的头结点。
- `while cur_node:`:遍历整个链表,直到当前节点为空。
- `print(cur_node.val, end=' ')`:输出当前节点的值。
- `cur_node = cur_node.next`:将当前节点指向下一个节点。
- `print()`:输出一个空行。
- `def reverse_traverse_doubly_linked_list(self, head):`:定义一个逆序遍历双向链表的函数。
- `cur_node = head`:将当前节点指向链表的头结点。
- `while cur_node.next:`:找到链表的尾结点。
- `cur_node = cur_node.next`:将当前节点指向下一个节点。
- `while cur_node:`:逆序遍历整个链表,直到当前节点为空。
- `print(cur_node.val, end=' ')`:输出当前节点的值。
- `cur_node = cur_node.prev`:将当前节点指向上一个节点。
- `print()`:输出一个空行。
阅读全文
相关推荐
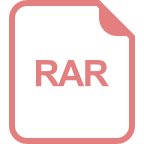
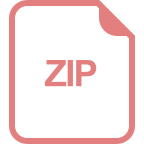
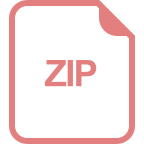












