完成自定义链表结构 1.添加数据 2.删除数据 3.修改数据 4.获得数据个数 5.通过索引获得数据 6.查询链表数据是否存在
时间: 2024-03-10 10:47:20 浏览: 17
好的,我可以帮你实现自定义链表结构。以下是链表节点的定义和链表类的实现,具体操作的实现可以根据你的需要进行修改:
```python
# 链表节点类
class ListNode:
def __init__(self, val):
self.val = val
self.next = None
# 链表类
class LinkedList:
def __init__(self):
self.head = None
self.count = 0
# 添加数据
def add(self, val):
new_node = ListNode(val)
if not self.head:
self.head = new_node
else:
cur_node = self.head
while cur_node.next:
cur_node = cur_node.next
cur_node.next = new_node
self.count += 1
# 删除数据
def remove(self, val):
if not self.head:
return
if self.head.val == val:
self.head = self.head.next
self.count -= 1
return
prev_node = self.head
cur_node = self.head.next
while cur_node:
if cur_node.val == val:
prev_node.next = cur_node.next
self.count -= 1
return
prev_node = cur_node
cur_node = cur_node.next
# 修改数据
def modify(self, old_val, new_val):
cur_node = self.head
while cur_node:
if cur_node.val == old_val:
cur_node.val = new_val
return True
cur_node = cur_node.next
return False
# 获得数据个数
def get_count(self):
return self.count
# 通过索引获得数据
def get_val(self, index):
if index < 0 or index >= self.count:
return None
cur_node = self.head
for i in range(index):
cur_node = cur_node.next
return cur_node.val
# 查询链表数据是否存在
def search(self, val):
cur_node = self.head
while cur_node:
if cur_node.val == val:
return True
cur_node = cur_node.next
return False
```
以上就是一个简单的链表实现,你可以根据需要进行修改和扩展。
相关推荐
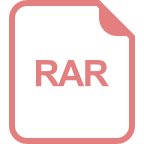
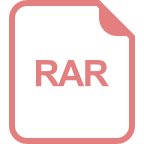
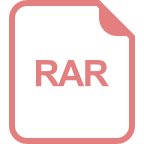














