python收集类函数的变量值
时间: 2025-01-02 21:37:00 浏览: 8
### 如何在 Python 中获取类方法内的变量值
为了实现从类方法内部捕获并访问局部变量,在 Python 中可以采用多种方式。以下是几种常见且有效的方法:
#### 方法一:通过返回值传递
最简单的方式是在方法内定义所需操作并将结果作为返回值传出。
```python
class ExampleClass:
def method_with_variable(self):
variable_inside_method = "Value inside the method"
return variable_inside_method
example_instance = ExampleClass()
value_from_method = example_instance.method_with_variable() # 获取到方法里的变量值[^1]
print(value_from_method)
```
#### 方法二:利用实例属性存储临时数据
如果希望在整个对象生命周期中保持某些状态,则可以在初始化时创建相应的成员变量用于保存这些信息;之后任何地方调用此对象的方法都可以修改或查询这个成员变量的内容。
```python
class ExampleClassWithAttribute:
def __init__(self):
self.stored_value = None
def set_stored_value_in_method(self):
local_var = "Local value to be stored"
self.stored_value = local_var
instance = ExampleClassWithAttribute()
instance.set_stored_value_in_method()
accessed_value = instance.stored_value # 访问之前设置好的变量值
print(accessed_value)
```
#### 方法三:借助闭包特性捕捉外部作用域中的名称空间
当涉及到更复杂的情况比如装饰器模式下,可以通过闭包机制来间接达到目的——即让外层函数记住其执行上下文中的一些特定绑定关系以便后续使用。
```python
def outer_function():
captured_local = 'Captured by closure'
class InnerClass:
@staticmethod
def get_captured_value():
nonlocal captured_local
return captured_local
return InnerClass.get_captured_value()
get_value_func = outer_function()
retrieved_value = get_value_func() # 使用闭包获得封闭函数里定义的变量值
print(retrieved_value)
```
以上三种方案分别适用于不同场景下的需求,可以根据实际情况灵活选用最适合的一种或者组合起来解决问题。
阅读全文
相关推荐














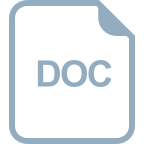



