UnicodeDecodeError: 'utf-8' codec can't decode byte 0xcf in position 964: invalid continuation byte
时间: 2023-10-29 07:57:37 浏览: 83
这个错误通常表示在尝试将字节序列解码为UTF-8字符串时遇到了无效的字节。可能是因为字节序列包含了不符合UTF-8编码规则的字节。
要解决这个问题,你可以尝试以下几个步骤:
1. 检查数据源:确保你的数据源是按照UTF-8编码保存的。如果不是,尝试使用正确的编码格式进行解码。
2. 处理无效字节:有时候,数据中可能包含一些无效或损坏的字节。你可以尝试使用错误处理策略来处理这些字节,例如忽略或替换它们。你可以在解码函数中指定错误处理策略,例如:
```python
data.decode('utf-8', errors='ignore') # 忽略无效字节
data.decode('utf-8', errors='replace') # 替换无效字节为特殊字符
```
3. 使用其他编码:如果以上方法无法解决问题,你可以尝试使用其他编码格式进行解码。常见的编码格式包括GBK、UTF-16等。根据实际情况选择适合的编码格式进行尝试。
请注意,如果你在处理文件时遇到此错误,确保以二进制模式打开文件,然后再进行解码操作。例如:
```python
with open('file.txt', 'rb') as file:
data = file.read().decode('utf-8')
```
这样可以确保文件内容以字节序列的形式读取,然后再进行解码操作。
希望这些方法能够帮助你解决问题!如果还有其他问题,请随时提问。
相关问题
UnicodeDecodeError: 'utf-8' codec can't decode byte 0xcf in position 0: invalid continuation byte
这个错误通常是因为你的代码尝试使用 UTF-8 编码格式读取一个文件,但是这个文件实际上不是以 UTF-8 格式编码的。这种情况下,Python 会尝试将文件内容解码为 UTF-8 格式,但是由于文件中包含了无效的字节,导致解码失败,从而抛出 UnicodeDecodeError 异常。
要解决这个问题,你需要确定文件实际上使用了哪种编码格式。你可以尝试使用一些工具来检测文件的编码格式,例如 chardet 或者 file命令。一旦你确定了文件的编码格式,就可以使用正确的编码格式打开文件进行读取,例如:
```
with open('file.txt', 'r', encoding='gbk') as f:
content = f.read()
```
其中 `gbk` 是文件实际上使用的编码格式。
UnicodeDecodeError: 'utf-8' codec can't decode byte 0xcf in position 17: invalid continuation byte
This error occurs when Python tries to decode a byte sequence into a string using the UTF-8 codec, but encounters a byte that cannot be decoded. In this case, the byte at position 17 has the value 0xcf, which is not a valid continuation byte in UTF-8 encoding.
To fix this error, you can try the following:
1. Check that the input data is actually encoded in UTF-8. If it is not, you may need to use a different codec to decode it.
2. If the input data is supposed to be in UTF-8, then the issue may be with the byte sequence itself. Try removing or replacing the problematic byte(s) and see if that resolves the issue.
3. If you are working with files, make sure you are opening them in binary mode (i.e. with the 'b' flag), as text mode may cause encoding issues.
4. You can also try using the 'errors' parameter when decoding, which will tell Python how to handle errors during decoding. For example, you can use 'ignore' to skip invalid bytes, or 'replace' to replace them with a special character.
Example:
```python
data = b'some byte string with \xcf invalid byte'
try:
decoded_data = data.decode('utf-8', errors='ignore')
except UnicodeDecodeError as e:
print(f"Error: {e}")
else:
print(decoded_data)
```
Output:
```
some byte string with invalid byte
```
阅读全文
相关推荐
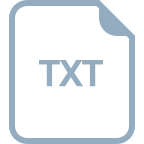
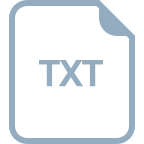














