c语言欧几里得拓展算法
时间: 2023-11-07 20:04:30 浏览: 129
欧几里德拓展算法是一种用于求解两个正整数最大公约数以及计算模逆元的算法。下面是C语言中的欧几里德拓展算法的描述:
```c
#include <stdio.h>
// 计算最大公约数并求解模逆元
int extendedEuclidean(int a, int b, int *x, int *y)
{
if (b == 0)
{
*x = 1;
*y = 0;
return a;
}
int x1, y1;
int gcd = extendedEuclidean(b, a % b, &x1, &y1);
*x = y1;
*y = x1 - a / b * y1;
return gcd;
}
int main()
{
int a, b;
printf("请输入两个正整数a和b:");
scanf("%d %d", &a, &b);
int x, y;
int gcd = extendedEuclidean(a, b, &x, &y);
printf("最大公约数为:%d\n", gcd);
printf("%d模%d的乘法逆元为:%d\n", a, b, x);
return 0;
}
```
相关问题
用C语言完成 拓展欧几里得算法过程,完成求最大公约数和求裴蜀对的操作并把拓展欧几里得算法写成一个函数
在C语言中,我们可以编写一个函数来实现拓展欧几里得算法,它不仅可以计算出两个整数的最大公约数,还能同时找到满足一定条件的一组解x和y(x * a + y * b = gcd(a, b))。这是利用了中国剩余定理的一个特殊情况。以下是基本的函数实现:
```c
#include <stdio.h>
// 定义最大公约数和模逆元的结构体
typedef struct {
int gcd; // 最大公约数
int x; // x的值
int y; // y的值
} ExtendedEuclideanResult;
ExtendedEuclideanResult extended_euclidean(int a, int b) {
if (b == 0) {
ExtendedEuclideanResult result;
result.gcd = a;
result.x = 1;
result.y = 0;
return result;
} else {
ExtendedEuclideanResult small_result = extended_euclidean(b, a % b);
int temp_x = small_result.y;
int temp_y = small_result.x - (a / b) * small_result.y;
ExtendedEuclideanResult result;
result.gcd = small_result.gcd;
result.x = temp_y;
result.y = temp_x;
return result;
}
}
int main() {
int a, b;
printf("请输入两个整数:");
scanf("%d%d", &a, &b);
ExtendedEuclideanResult result = extended_euclidean(a, b);
printf("最大公约数:%d\n", result.gcd);
printf("裴蜀对解(x, y): (%d, %d)\n", result.x, result.y);
return 0;
}
```
在这个函数中,我们首先检查是否b为0,如果是则直接返回结果。如果b不为0,递归地调用自身,直到b为0。最后根据公式计算x和y的值。
阅读全文
相关推荐
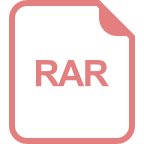



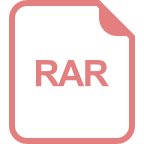
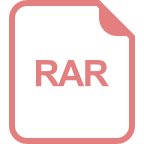







