class hello{void temp()}; class world{ static hello * hel;}调用temp函数 在class world{} static hello * hel;就相当于定义了hello实例了吗
时间: 2024-09-24 11:23:19 浏览: 31
在Java中,`class hello{void temp();}` 定义了一个名为 `hello` 的类,其中有一个无参的方法 `temp()`。而 `class world{static hello * hel;}` 中的 `hel` 是一个指向 `hello` 类的指针,它是静态的,意味着它属于 `world` 类而不是具体的 `hello` 实例。
如果你想要调用 `temp` 函数,通常需要创建一个 `hello` 类的实例并调用该方法。由于 `hel` 是一个静态成员变量,你可以直接通过 `world` 类来访问它,但不会自动创建一个新的 `hello` 对象。如果你想通过 `hel` 调用 `temp()`,你需要先初始化它:
```java
// 假设我们有一个构造函数用于创建hello对象
public hello() {
this.temp();
}
world w = new world();
w.hel.new hello().temp(); // 如果hel尚未初始化,这会抛出NullPointerException
```
这里假设 `hello` 类有一个无参数的构造函数,以便于创建新的实例。如果 `hel` 没有初始化,试图通过它调用 `temp()` 会抛出 `NullPointerException`,因为 Java 强调对象在使用前必须被正确地初始化。
相关问题
用动态分配实现字符串循环左移,例如: 输入 hello world! 3 输出 lo world!hel
在动态内存分配中,我们可以使用字符数组或字符串来实现字符串的循环左移。这里是一个简单的Python示例:
```python
def cyclic_left_shift(s, shift):
# 获取字符串长度
n = len(s)
# 验证移动位数是否大于等于零且不大于字符串长度
if shift >= 0 and shift < n:
# 动态分配新的字符串,创建一个空串用于存放结果
new_str = ['\0'] * (n + shift)
# 将原字符串的最后shift位复制到新字符串的开始位置
for i in range(shift):
new_str[i] = s[n - i - 1]
# 将剩余部分连接到新字符串的末尾
for i in range(n - shift):
new_str[i + shift] = s[i]
# 返回处理后的字符串
return ''.join(new_str)
else:
print("Invalid shift value.")
return None
# 示例输入
s = "hello world!"
shift = 3
result = cyclic_left_shift(s, shift)
print(f"Output: {result}")
C语言用动态分配实现字符串循环左移,例如: 输入 hello world! 3 输出 lo world!hel
在C语言中,动态分配内存通常通过`malloc()`函数来实现字符串的循环左移操作。对于给定的字符串和位移量,可以按以下步骤操作:
1. 首先,计算新的字符串长度,原字符串加上位移后的字符数减去原始字符串的首字符(因为循环左移会跳过第一个字符)。
2. 使用`malloc()`为新字符串分配足够的空间,假设新长度为`new_len`。
3. 将原字符串中的每个字符复制到新位置,如果需要的话,跳过已移动过的部分。
4. 如果位移超过字符串长度,将剩余部分添加到新字符串的开始,保持循环效果。
以下是一个简单的示例代码片段,演示如何实现这一功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void left_rotate(char* str, int shift) {
int len = strlen(str);
shift %= len; // 转移到的有效范围
char* new_str = (char*)malloc((len + shift) * sizeof(char)); // 动态分配空间
if (!new_str) {
printf("Memory allocation failed.\n");
return;
}
// 将原字符串复制到新位置并插入循环部分
for (int i = 0; i < len; ++i) {
new_str[(i + shift) % len] = str[i];
}
// 将剩余部分连接到新字符串开头
if (shift > 0) {
strcat(new_str, str);
}
strcpy(str, new_str); // 更新原字符串指向新字符串
free(new_str); // 释放旧字符串内存
}
int main() {
char input[] = "hello world!";
int shift = 3;
left_rotate(input, shift);
printf("%s\n", input); // 输出结果
阅读全文
相关推荐
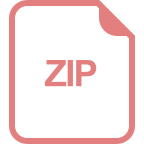
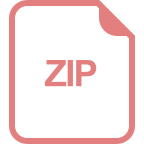
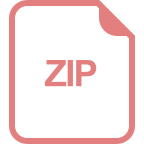
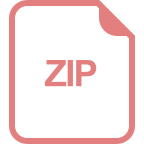










