设计一个算法,使用C语言实现:使用结构体和数组存储线性表结构,实现线性表的以 下操作:初始化、求长度、添加新节点、输出所有节点、任意位置插入新节点、删除指 定位置节点、按序号查找节点、按输入关键字查找序号。
时间: 2024-09-18 22:03:38 浏览: 27
设计这样一个C语言程序,我们可以创建一个名为`ListNode`的结构体表示线性表的节点,并使用一个动态数组`nodeArray`存储节点。以下是关键部分的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 结构体表示节点
typedef struct ListNode {
int data;
struct ListNode* next;
} Node;
// 动态数组用于存储节点
Node** nodeArray;
int size = 0, capacity;
// 初始化函数
void initList() {
if (size == capacity) {
capacity *= 2; // 当容量不足时扩大一倍
nodeArray = realloc(nodeArray, sizeof(Node*) * capacity);
}
nodeArray[size] = NULL;
size++;
}
// 求长度
int length() {
return size;
}
// 添加新节点
void addNode(int value) {
Node* newNode = malloc(sizeof(Node));
newNode->data = value;
newNode->next = nodeArray[size]; // 将新节点添加到链表尾部
nodeArray[size++] = newNode;
}
// 输出所有节点
void printList() {
for (int i = 0; i < size; i++) {
printf("%d ", nodeArray[i]->data);
}
printf("\n");
}
// 在指定位置插入新节点
void insertAt(int position, int value) {
if (position <= size) {
Node* newNode = malloc(sizeof(Node));
newNode->data = value;
newNode->next = nodeArray[position];
for (int i = size - 1; i >= position; i--) {
nodeArray[i + 1] = nodeArray[i];
}
nodeArray[position] = newNode;
size++;
} else {
printf("Invalid position.\n");
}
}
// 删除指定位置的节点
void deleteNode(int position) {
if (position < size) {
for (int i = position; i < size - 1; i++) {
nodeArray[i] = nodeArray[i + 1];
}
free(nodeArray[size - 1]);
size--;
} else {
printf("Invalid position.\n");
}
}
// 查找节点(按序号)
int findNodeByIndex(int index) {
if (index >= 0 && index < size) {
return nodeArray[index]->data;
} else {
printf("Index out of range.\n");
return -1;
}
}
// 按关键字查找序号(这里假设关键字是一个整数,实际应用需调整)
int findNodeByKey(int key) {
for (int i = 0; i < size; i++) {
if (nodeArray[i]->data == key) {
return i;
}
}
return -1; // 如果找不到,返回-1
}
// 示例如何使用这个结构
int main() {
nodeArray = malloc(sizeof(Node*) * 5); // 初始容量
initList();
// 使用addNode(), insertAt(), etc...
return 0;
}
```
相关推荐
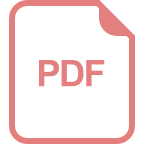
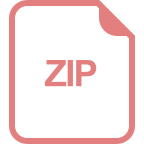

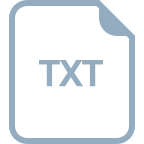
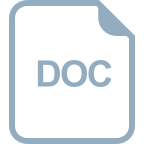
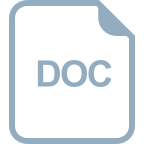
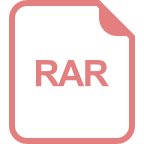
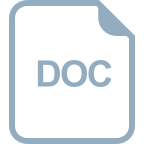
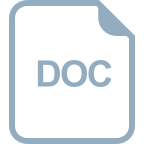
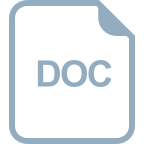
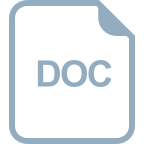
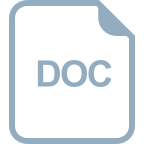
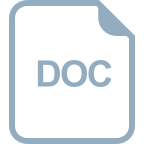
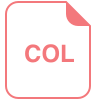
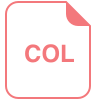
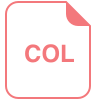
