设计一个Car类,它的数据成员要能描述一辆汽车的品牌、型号、出厂年份和价格,成员函数包括提供合适的途径来访问数据成员,在main()函数中定义类的对象并调用相应成员函数。
时间: 2024-11-03 22:15:19 浏览: 24
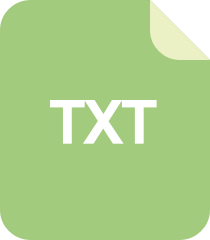
设计一个Car类
设计一个名为`Car`的类,我们可以这样实现:
```cpp
class Car {
private:
std::string brand; // 汽车品牌
std::string model; // 汽车型号
int year_of_manufacture; // 出厂年份
double price; // 价格
public:
// 构造函数用于初始化数据成员
Car(const std::string& brand, const std::string& model, int year, double price)
: brand(brand), model(model), year_of_manufacture(year), price(price) {}
// 成员函数:获取数据成员值
std::string getBrand() const { return brand; }
std::string getModel() const { return model; }
int getYearOfManufacture() const { return year_of_manufacture; }
double getPrice() const { return price; }
// 成员函数:设置数据成员值(若需要)
void setBrand(const std::string& new_brand) { brand = new_brand; }
void setModel(const std::string& new_model) { model = new_model; }
void setPrice(double new_price) { price = new_price; }
// 主函数中创建对象并调用成员函数示例
static void mainExample() {
Car myCar("Toyota", "Camry", 2022, 25000.0);
std::cout << "Brand: " << myCar.getBrand() << std::endl;
std::cout << "Model: " << myCar.getModel() << std::endl;
std::cout << "Year of manufacture: " << myCar.getYearOfManufacture() << std::endl;
std::cout << "Price: $" << myCar.getPrice() << std::endl;
// 调整属性
myCar.setPrice(27000.0);
std::cout << "Updated price: $" << myCar.getPrice() << std::endl;
}
};
```
在这个例子中,我们定义了`Car`类的数据成员和相应的getter和setter方法,以便于访问和修改这些数据。`mainExample()`函数展示了如何创建`Car`对象并调用这些功能。
阅读全文
相关推荐
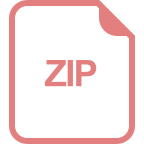
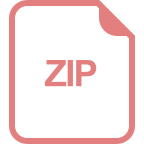















